简单的线程通信,一个线程对数字进行增加操作、另一个线程对线程进行减少操作.
简单解释:线程A对数字进行减少操作,但不会一直让这个数字减少下去.当减少到设定的条件,让其等待,通知其他线程获得该资源.
package com.ProductAndCustomer;
/**
* 线程之间的通信,生产者和消费者问题
*/
public class Product {
public static void main(String[] args) {
Data data = new Data();
new Thread(()->{
for (int i = 0; i < 10; i++) {
try {
data.increment();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
},"A").start();
new Thread(()->{
for (int i = 0; i < 10; i++) {
try {
data.decrement();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
},"B").start();
}
}
//等待,业务,通知
class Data{
//资源类
private int num = 0;
//增加操作
public synchronized void increment() throws InterruptedException {
if(num != 0){
//等待
this.wait();
}
num ++;
System.out.println(Thread.currentThread().getName()+"=>"+num);
//通知其他线程,+1结束
this.notifyAll();
}
//减少操作
public synchronized void decrement() throws InterruptedException {
if(num == 0){
//等待
this.wait();
}
num --;
System.out.println(Thread.currentThread().getName()+"=>"+num);
//通知其他线程,-1结束
this.notifyAll();
}
}
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
- 14.
- 15.
- 16.
- 17.
- 18.
- 19.
- 20.
- 21.
- 22.
- 23.
- 24.
- 25.
- 26.
- 27.
- 28.
- 29.
- 30.
- 31.
- 32.
- 33.
- 34.
- 35.
- 36.
- 37.
- 38.
- 39.
- 40.
- 41.
- 42.
- 43.
- 44.
- 45.
- 46.
- 47.
- 48.
- 49.
- 50.
- 51.
- 52.
- 53.
- 54.
- 55.
- 56.
- 57.
- 58.
- 59.
- 60.
- 61.
- 62.
测试

在多几个线程
package com.ProductAndCustomer;
/**
* 线程之间的通信,生产者和消费者问题
*/
public class Product {
public static void main(String[] args) {
Data data = new Data();
new Thread(()->{
for (int i = 0; i < 10; i++) {
try {
data.increment();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
},"A").start();
new Thread(()->{
for (int i = 0; i < 10; i++) {
try {
data.decrement();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
},"B").start();
new Thread(()->{
for (int i = 0; i < 10; i++) {
try {
data.decrement();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
},"C").start();
new Thread(()->{
for (int i = 0; i < 10; i++) {
try {
data.decrement();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
},"D").start();
}
}
//等待,业务,通知
class Data{
//资源类
private int num = 0;
//增加操作
public synchronized void increment() throws InterruptedException {
if(num != 0){
//等待
this.wait();
}
num ++;
System.out.println(Thread.currentThread().getName()+"=>"+num);
//通知其他线程,+1结束
this.notifyAll();
}
//减少操作
public synchronized void decrement() throws InterruptedException {
if(num == 0){
//等待
this.wait();
}
num --;
System.out.println(Thread.currentThread().getName()+"=>"+num);
//通知其他线程,-1结束
this.notifyAll();
}
}
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
- 14.
- 15.
- 16.
- 17.
- 18.
- 19.
- 20.
- 21.
- 22.
- 23.
- 24.
- 25.
- 26.
- 27.
- 28.
- 29.
- 30.
- 31.
- 32.
- 33.
- 34.
- 35.
- 36.
- 37.
- 38.
- 39.
- 40.
- 41.
- 42.
- 43.
- 44.
- 45.
- 46.
- 47.
- 48.
- 49.
- 50.
- 51.
- 52.
- 53.
- 54.
- 55.
- 56.
- 57.
- 58.
- 59.
- 60.
- 61.
- 62.
- 63.
- 64.
- 65.
- 66.
- 67.
- 68.
- 69.
- 70.
- 71.
- 72.
- 73.
- 74.
- 75.
- 76.
- 77.
- 78.
- 79.
- 80.
- 81.
- 82.
- 83.
- 84.
测试结果
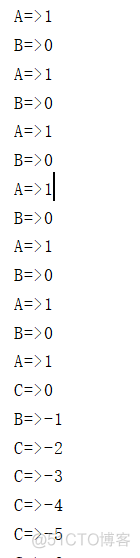
这里会出现虚假唤醒
查看开发文档可知、需要修改判断语句 if修改为while
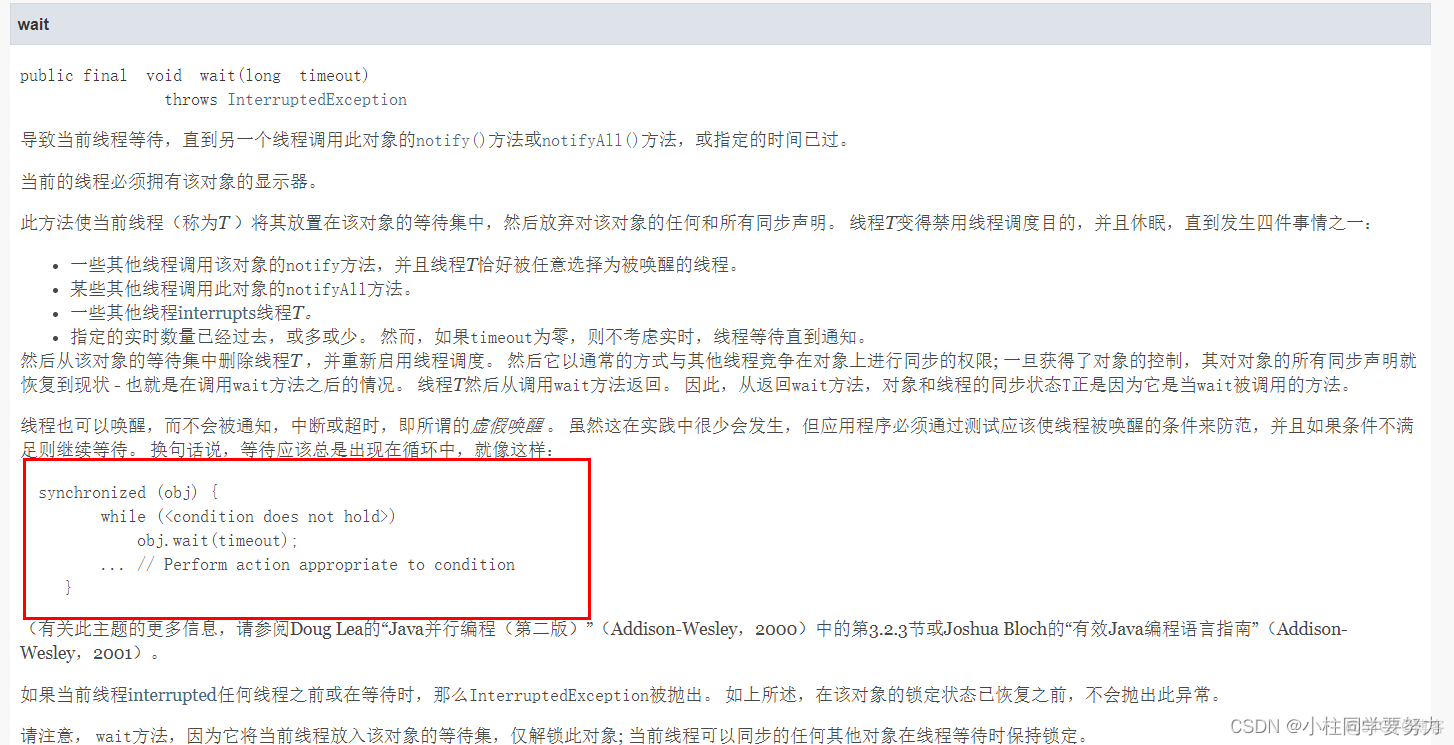
修改后的代码
package com.ProductAndCustomer;
/**
* 线程之间的通信,生产者和消费者问题
*/
public class Product {
public static void main(String[] args) {
Data data = new Data();
new Thread(()->{
for (int i = 0; i < 5; i++) {
try {
data.increment();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
},"A").start();
new Thread(()->{
for (int i = 0; i < 5; i++) {
try {
data.decrement();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
},"B").start();
new Thread(()->{
for (int i = 0; i < 5; i++) {
try {
data.increment();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
},"C").start();
new Thread(()->{
for (int i = 0; i < 5; i++) {
try {
data.decrement();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
},"D").start();
}
}
//等待,业务,通知
class Data{
//资源类
private int num = 0;
//增加操作
public synchronized void increment() throws InterruptedException {
while(num != 0){
//等待
this.wait();
}
num ++;
System.out.println(Thread.currentThread().getName()+"=>"+num);
//通知其他线程,+1结束
this.notifyAll();
}
//减少操作
public synchronized void decrement() throws InterruptedException {
while(num == 0){
//等待
this.wait();
}
num --;
System.out.println(Thread.currentThread().getName()+"=>"+num);
//通知其他线程,-1结束
this.notifyAll();
}
}
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
- 14.
- 15.
- 16.
- 17.
- 18.
- 19.
- 20.
- 21.
- 22.
- 23.
- 24.
- 25.
- 26.
- 27.
- 28.
- 29.
- 30.
- 31.
- 32.
- 33.
- 34.
- 35.
- 36.
- 37.
- 38.
- 39.
- 40.
- 41.
- 42.
- 43.
- 44.
- 45.
- 46.
- 47.
- 48.
- 49.
- 50.
- 51.
- 52.
- 53.
- 54.
- 55.
- 56.
- 57.
- 58.
- 59.
- 60.
- 61.
- 62.
- 63.
- 64.
- 65.
- 66.
- 67.
- 68.
- 69.
- 70.
- 71.
- 72.
- 73.
- 74.
- 75.
- 76.
- 77.
- 78.
- 79.
- 80.
- 81.
- 82.
- 83.
- 84.
测试结果
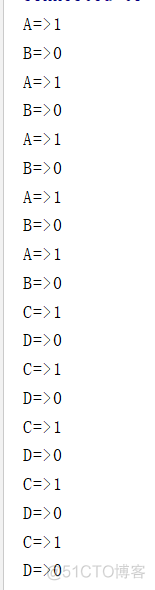
原网站版权声明
本文为[51CTO]所创,转载请带上原文链接,感谢
https://yzsam.com/2022/216/202208040856289145.html