当前位置:网站首页>Enumeration general interface & enumeration usage specification
Enumeration general interface & enumeration usage specification
2022-07-07 03:25:00 【InfoQ】
- The field value in the data table is the field of finite sequence , Corresponding to the specific enumeration in the program . Try to use varchar replace int( or tinyint). Beyond all doubt , Letter combinations are always better than 0、1、2、3 Such numbers are easy to recognize .
- If the data table field has corresponding enumeration , be , The enumeration class name should be marked on the field annotation , Facilitate program traceability .
- Enumeration generally has two parts , One is to enumerate item values , One is enumeration description . that , How are these two attributes named ? code and desc? still value and desc? still key and value? This article focuses on this problem .
@Getter
@AllArgsConstructor
public enum LevelEnum {
FIRST("FIRST", " Class A "),
SECOND("SECOND", " second level "),
THIRD("THIRD", " Level three ");
private String code;
private String value;
public static LevelEnum getBeanByCode(String code) {
LevelEnum[] statusEnums = LevelEnum.values();
for (LevelEnum v : statusEnums) {
if (v.getCode().equals(code)) {
return v;
}
}
return null;
}
}
@Getter
@AllArgsConstructor
public enum OrderStatusEnum {
INIT("INIT", " initial "),
ACCOUNTING("ACCOUNTING", " In bookkeeping "),
SUCCESS("SUCCESS", " Payment succeeded "),
FAILED("FAILED", " Payment failed ");
private String key;
private String description;
public static OrderStatusEnum getBeanByCode(String code) {
OrderStatusEnum[] values = OrderStatusEnum.values();
for (OrderStatusEnum v : values) {
if (v.getKey().equals(code)) {
return v;
}
}
return null;
}
}
@AllArgsConstructor
public enum ProductEnum {
BOSSKG("BOSS starts "),
HUICHUXING(" Benefit travel "),
SICHEBANGONG(" Private car office "),
YOUFU(" Youfu "),
UNKNOWN(" Unknown "),
;
private String description;
private String getCode(){
return this.toString();
}
public String getDescription() {
return description;
}
public static ProductEnum getBean(String value) {
ProductEnum[] values = ProductEnum.values();
for(ProductEnum temp : values){
if(temp.getCode().equals(value)){
return temp;
}
}
return ProductEnum.UNKNOWN;
}
}
@Getter
@AllArgsConstructor
public enum SeasonEnum {
SPRING(1, " In the spring "),
SUMMER(2, " In the summer "),
AUTUMN(3, " autumn "),
WINTER(4, " In the winter ");
private int code;
private String description;
public static SeasonEnum getBeanByCode(Integer code) {
if (null == code) return null;
SeasonEnum[] values = SeasonEnum.values();
for (SeasonEnum temp : values) {
if (temp.getCode() == code) {
return temp;
}
}
return null;
}
}
/**
* If the enumeration name is different from the actual value , Be sure to rewrite getKey Method
* Enumeration definition specification : Remember to capitalize enumeration names , The description should be as clear as possible , Don't misspell , Please check carefully
* for example :
* MONDAY(" Monday "),
* TUESDAY(" Tuesday ")
*
* @author shaozhengmao
* @create 2021-06-21 10:18 In the morning
*/
public interface EnumAbility<T> {
/**
* Return the actual value of enumeration
* @return
*/
T getCode();
/**
* Return enumeration description
*
* @return Enumeration description
*/
String getDescription();
/**
* Compare whether the current enumeration object is consistent with the passed in enumeration value (String Types ignore case )
* Whether the current enumeration item matches the value passed in from the far end ( such as : Field value of database 、rpc The parameter value passed in )
*
* @param enumCode enumeration code
* @return match
*/
default boolean codeEquals(T enumCode) {
if (enumCode == null) return false;
if (enumCode instanceof String) {
return ((String) enumCode).equalsIgnoreCase((String) getCode());
} else {
return Objects.equals(this.getCode(), enumCode);
}
}
/**
* Compare whether the two enumeration items are identical (==)
*
* @param anotherEnum enumeration
* @return Are they the same?
*/
default boolean equals(EnumAbility<T> anotherEnum) {
return this == anotherEnum;
}
}
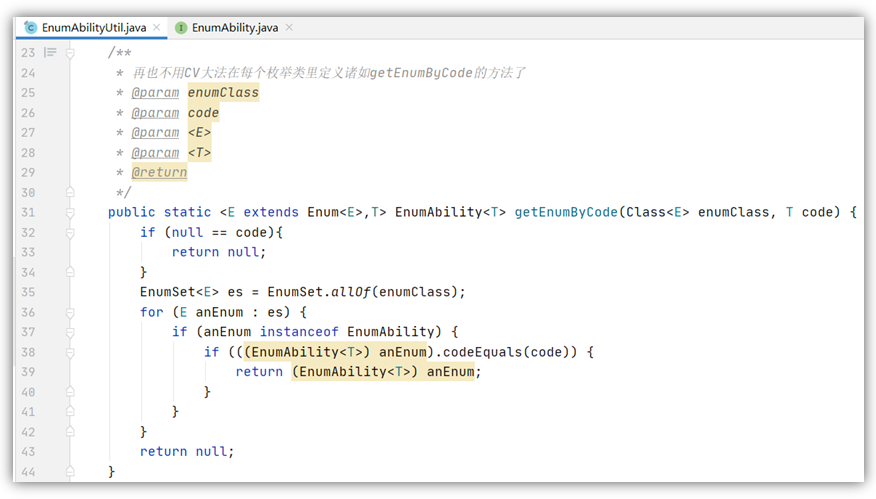
@Getter
@AllArgsConstructor
public enum LevelEnum implements EnumAbility<String> {
FIRST("FIRST", " Class A "),
SECOND("SECOND", " second level "),
THIRD("THIRD", " Level three ");
private String code;
private String value;
@Override
public String getDescription() {
return value;
}
/**
* 2021-12-18 23:00 zhanggz: There is ambiguity in this method , Please use {@link #getDescription()}
* @return
*/
@Deprecated
public String getValue() {
return value;
}
public static LevelEnum getBeanByCode(String code) {
return (LevelEnum) EnumAbilityUtil.getEnumByCode(LevelEnum.class, code);
}
}
@Getter
@AllArgsConstructor
public enum OrderStatusEnum implements EnumAbility<String> {
INIT("INIT", " initial "),
ACCOUNTING("ACCOUNTING", " In bookkeeping "),
SUCCESS("SUCCESS", " Payment succeeded "),
FAILED("FAILED", " Payment failed ");
private String key;
private String description;
@Override
public String getCode() {
return key;
}
/**
* 2021-12-18 23:00 zhanggz: There is ambiguity in this method , Please use {@link #getCode()}
* @return
*/
@Deprecated
public String getKey() {
return key;
}
public static OrderStatusEnum getBeanByCode(String code) {
return (OrderStatusEnum) EnumAbilityUtil.getEnumByCode(OrderStatusEnum.class, code);
}
}
@AllArgsConstructor
public enum ProductEnum implements EnumAbility<String> {
BOSSKG("BOSS starts "),
HUICHUXING(" Benefit travel "),
SICHEBANGONG(" Private car office "),
YOUFU(" Youfu "),
UNKNOWN(" Unknown "),
;
private String description;
@Override
private String getCode(){
return this.toString();
}
@Override
public String getDescription() {
return description;
}
public static ProductEnum getBean(String code) {
return (ProductEnum) EnumAbilityUtil.getEnumByCode(ProductEnum.class, code);
}
}
@Getter
@AllArgsConstructor
public enum SeasonEnum implements EnumAbility<Integer> {
SPRING(1, " In the spring "),
SUMMER(2, " In the summer "),
AUTUMN(3, " autumn "),
WINTER(4, " In the winter ");
private Integer code;
private String description;
public static SeasonEnum getBeanByCode(Integer code) {
if (null == code) return null;
return (SeasonEnum) EnumAbilityUtil.getEnumByCode(SeasonEnum.class, code);
}
}
边栏推荐
- 腾讯云原生数据库TDSQL-C入选信通院《云原生产品目录》
- 23.(arcgis api for js篇)arcgis api for js椭圆采集(SketchViewModel)
- Mathematical induction and recursion
- 腾讯云原生数据库TDSQL-C入选信通院《云原生产品目录》
- Lavel PHP artisan automatically generates a complete set of model+migrate+controller commands
- Appx代码签名指南
- Jerry's question about DAC output power [chapter]
- 华为小米互“抄作业”
- Domcontentloaded and window onload
- Jericho is in non Bluetooth mode. Do not jump back to Bluetooth mode when connecting the mobile phone [chapter]
猜你喜欢
25.(arcgis api for js篇)arcgis api for js线修改线编辑(SketchViewModel)
Flink task exit process and failover mechanism
Decoration design enterprise website management system source code (including mobile source code)
leetcode
如何自定义Latex停止运行的快捷键
Leetcode-02 (linked list question)
Lavel PHP artisan automatically generates a complete set of model+migrate+controller commands
2022.6.28
Set WiFi automatic connection for raspberry pie
Create applet from 0
随机推荐
房费制——登录优化
An error in SQL tuning advisor ora-00600: internal error code, arguments: [kesqsmakebindvalue:obj]
netperf 而网络性能测量
Appx code signing Guide
Jerry's RTC clock development [chapter]
Don't you know the relationship between JSP and servlet?
杰理之FM 模式单声道或立体声选择设置【篇】
About Estimation Statistics
RestClould ETL 社区版六月精选问答
24.(arcgis api for js篇)arcgis api for js点修改点编辑(SketchViewModel)
Sorting operation partition, argpartition, sort, argsort in numpy
Graphical tools package yolov5 and generate executable files exe
Appx代码签名指南
Sub pixel corner detection opencv cornersubpix
Jericho is in non Bluetooth mode. Do not jump back to Bluetooth mode when connecting the mobile phone [chapter]
Principle of attention mechanism
Not All Points Are Equal Learning Highly Efficient Point-based Detectors for 3D LiDAR Point
Cryptography series: detailed explanation of online certificate status protocol OCSP
Mathematical induction and recursion
校招行测笔试-数量关系