当前位置:网站首页>uni-app 从零开始-基础模版(一)
uni-app 从零开始-基础模版(一)
2022-08-04 02:31:00 【吟秋知忆】
背景
由于公司需要开发多端小程序,使用原生开发,不仅增加了大量工作,还要维护多套代码(相同逻辑,重复开发),本着减少前期开发,降低后期优化迭代成本,通过市场调查对比后,最终打算在uniapp与taro中选择一种技术开发小程序。
技术对比
框架 | 技术栈 | 小程序 | H5 | App | 支持平台 | Star |
---|---|---|---|---|---|---|
uni-app | React/Vue | 微信/支付宝... | 支持 | Android/IOS | 14 | 36.9k+ |
taro | Vue | 微信/支付宝... | 支持 | ReactNative | 12+ | 31.4k+ |
「总结:」 uni-app 开发简单,小项目效率高,入门容易。 Taro3上手难度相对较高,需要拥有良好的编程基础,支付宝小程序有些东西需要自己兼容适配(其它端没用过)。
开发效率uni-app高,有自家的IDE(HBuilderX),编译调试打包一体化,对原生App开发体验友好; 技术栈方面Taro支持React/Vue,uni-app只支持Vue,从公司项目角度uni-app/Vue比较短平快,社区活跃度也比较高,更容易上手使用。
开始
使用Vue3/Vite创建项目基础模版,命令如下:
npx degit dcloudio/uni-preset-vue#vite-ts uniapp-applet
uni-ui
使用官方ui组件库,由官方同步维护,减少自己填坑
优点:高性能、全端、风格扩展、与uniCloud协作、与uni统计自动集成实现免打点、uni-ui符合全套DCloud组件规范
安装uni-ui
pnpm add @dcloudio/uni-ui
配置组件自动引入
在pages.json文件中配置easycom组件自动引入规则
{
"easycom": {
"autoscan": true,
"custom": {
"^uni-(.*)": "@dcloudio/uni-ui/lib/uni-$1/uni-$1.vue"
}
}
}
不需要导入直接使用
<tempate>
<uni-countdown :day="1" :hour="1" :minute="12" :second="40"></uni-countdown>
</template>
pinia
Vue 的状态存储库,它允许您跨组件、页面共享状态。了解更多查看pinia官方文档。
在stores/index.ts中:
import { createPinia } from 'pinia';
const store = createPinia();
export default store;
在stores/counter.ts中:
import { defineStore } from 'pinia';
export const useCounterStore = defineStore('counter', {
state: () => {
return { count: 0 };
},
actions: {
increment() {
this.count++;
},
},
});
在main.ts注册store:
import { createSSRApp } from "vue";
import store from '@/stores';
import App from "./App.vue";
export function createApp() {
const app = createSSRApp(App);
// 注册store
app.use(store);
return {
app,
};
}
在页面中使用:
<template>
<view class="content">
<view>counter:{{ counter.count }}</view>
<button @click="counter.increment">add</button>
</view>
</template>
<script setup lang="ts">
import { useCounterStore } from '@/stores/modules/counter';
const counter = useCounterStore();
</script>
图标库
阿里图标库:搭建自己的图标库,小程序使用base64
转换后的图标格式。
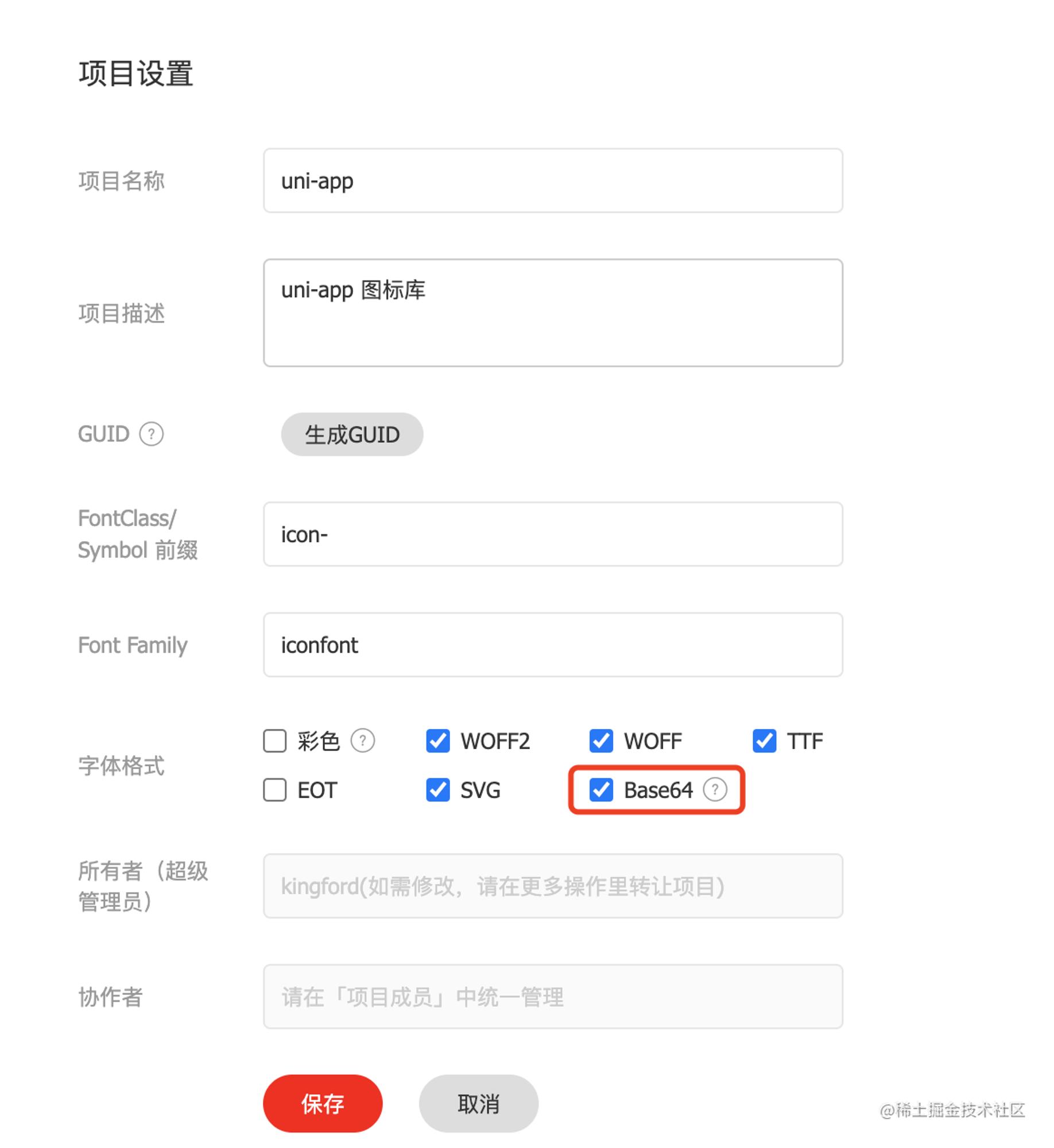
选择自己的图标,下载后复制iconfont.css的内容到自己的图标icon.scss样式文件; 删除引入本地的地址,只留base64转换后的地址; 在全局中App.vue或main.ts文件中引入图标样式。
<style lang="scss">
@import './styles/icon';
</style>
使用
<text class="iconfont icon-music"></text>
动画
animate css动画库
小技巧
背景图
本地背景图片推荐以~@
开头的绝对路径,背景图片建议小于40k,小于40k不支持本地背景平台编译自动转化为base64
格式。
.test2 {
background-image: url('[email protected]/static/logo.png');
}
easycom
easycom:只要组件安装在项目的
components
目录下,并符合components/组件名称/组件名称.vue
目录结构。就可以不用引用、注册,直接在页面中使用。
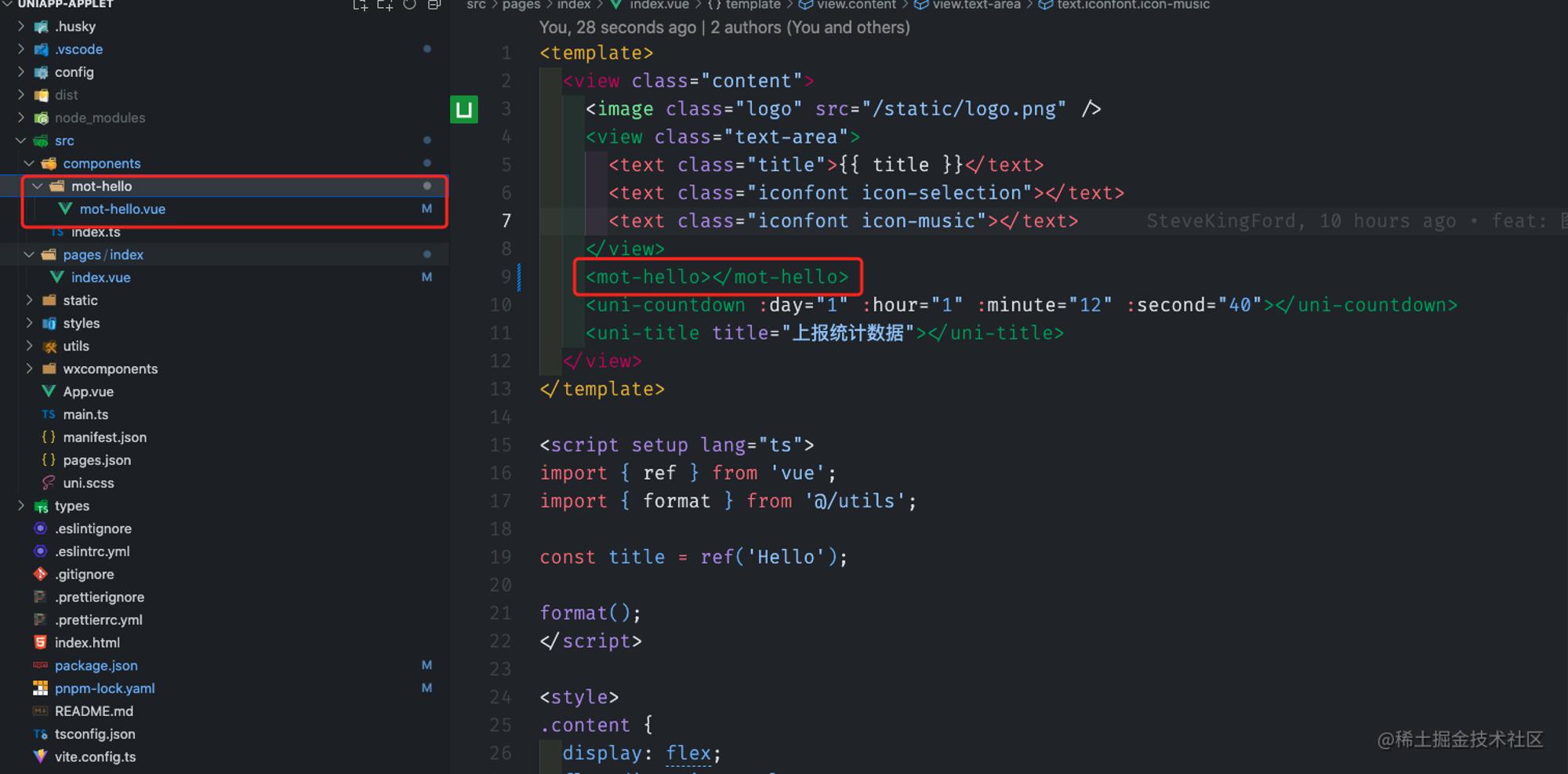
vite
优秀的vite插件推荐:
unocss原子化css vite-tsconfig-paths 配置别名映射 pinia-auto-refs 自动化storeToRefs(使用vuex可以忽略) unplugin-auto-import 自动导入 Vite、Webpack、Rollup 和 esbuild 的 API
更多配置查看vite官方文档
注意事项
vue3 响应式基于 Proxy
实现,不支持iOS9
和ie11
, 查看iOS and iPadOS usage。暂不支持新增的 Teleport
,Suspense
组件
推荐阅读
<script setup>
是在单文件组件 (SFC) 中使用组合式 API 的编译时语法糖。当同时使用 SFC 与组合式 API 时则推荐该语法。相比于普通的<script>
语法,它具有更多优势:
更少的样板内容,更简洁的代码。 能够使用纯 Typescript 声明 prop 和抛出事件。 更好的运行时性能 (其模板会被编译成同一作用域的渲染函数,没有任何的中间代理)。 更好的 IDE 类型推断性能 (减少语言服务器从代码中抽离类型的工作)。
defineProps
为了在声明
props
选项时获得完整的类型推断支持
<template>
<h1>{{ msg }}</h1>
</template>
<script setup lang="ts">
defineProps({
msg: String
})
</script>
defineEmits
为了在声明
emits
选项时获得完整的类型推断支持
<template>
<div @click="onClick">点我</div>
</template>
<script setup lang="ts">
const emit = defineEmits(['click', 'change', 'delete'])
const onClick = () => {
emit('click', 2)
}
</script>
defineExpose
使用
<script setup>
的组件是「默认关闭」的——即通过模板 ref 或者$parent
链获取到的组件的公开实例,「不会」暴露任何在<script setup>
中声明的绑定。
子组件child.vue暴露自己的属性
<template>
<div>子组件child.vue</div>
</template>
<script setup lang="ts">
import { ref } from 'vue'
const count = ref(123456)
defineExpose({
count
})
</script>
父组件获取子组件属性
<template>
<button @click="onClick">父组件</button>
<child ref="childRef"></child>
</template>
<script setup lang="ts">
import { ref } from 'vue'
import child from './components/child.vue'
const childRef = ref(null)
const onClick = () => {
console.log(childRef.value.count) // 123456
}
</script>
总结
本次基础模版分别引入:
uni-ui 官方ui库 pinia 状态管理工具 iconfont 图标库 animate css动画库 vite 优秀插件
关注我
需要免费兼职,每天五分钟,实现最美现金流;有感兴趣的,请联系我~
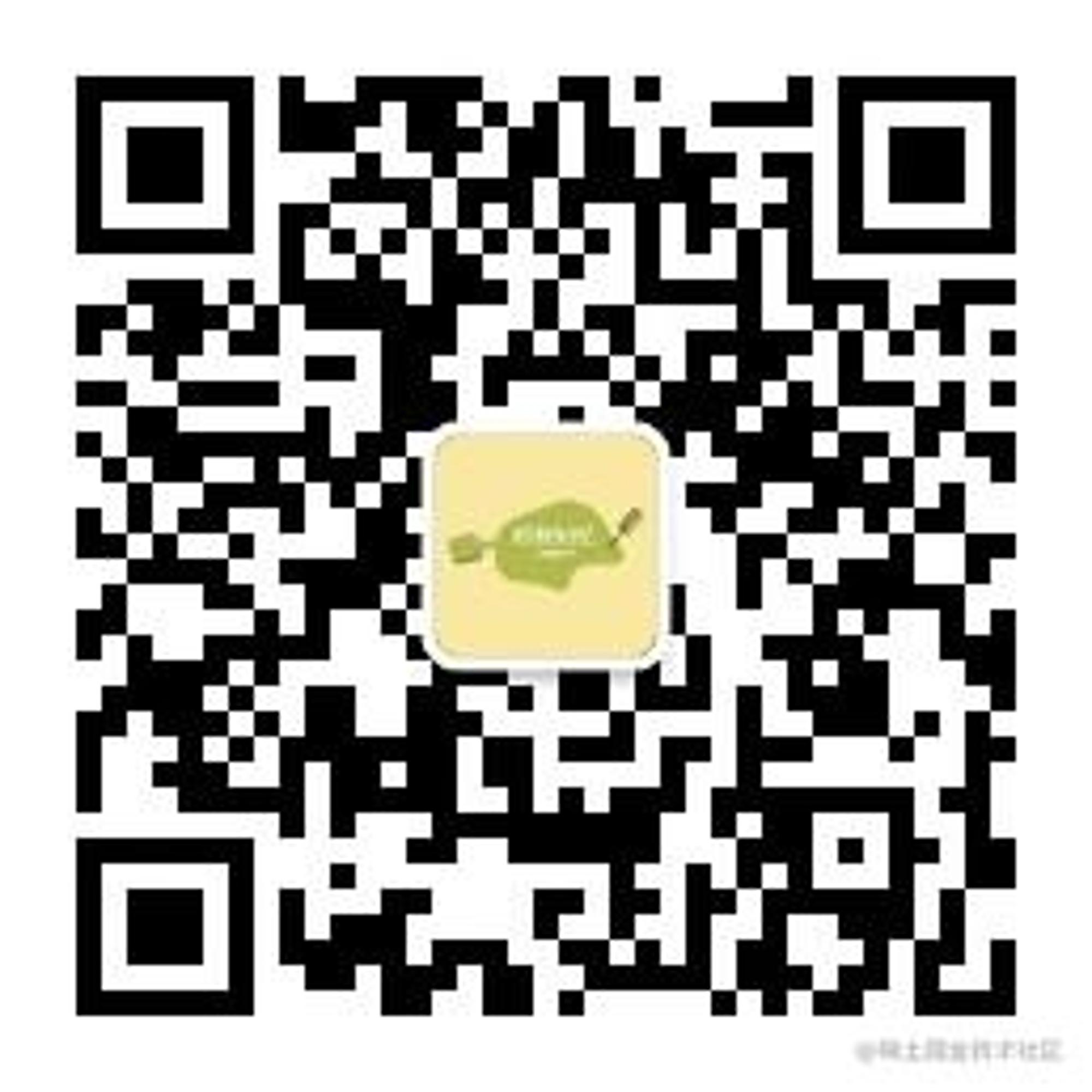
边栏推荐
- priority_queue元素为指针时,重载运算符失效
- In the season of going overseas, the localization of Internet tips for going overseas
- Continuing to invest in product research and development, Dingdong Maicai wins in supply chain investment
- 在更一般意义上验算移位距离和假设
- Multithreading JUC Learning Chapter 1 Steps to Create Multithreading
- 【Playwright测试教程】5分钟上手
- Flask Framework Beginner-05-Command Management Manager and Database Use
- Sky map coordinate system to Gaode coordinate system WGS84 to GCJ02
- SAP SD模块前台操作
- 2022G1工业锅炉司炉考试练习题及模拟考试
猜你喜欢
关联接口测试
5.scrapy中间件&分布式爬虫
实例035:设置输出颜色
ssh服务详解
Engineering drawing review questions (with answers)
架构实战营模块三作业
实例037:排序
2022年T电梯修理考题及答案
Flask Framework Beginner-06-Add, Delete, Modify and Check the Database
Continuing to invest in product research and development, Dingdong Maicai wins in supply chain investment
随机推荐
Example 039: Inserting elements into an ordered list
董明珠直播时冷脸离场,员工频犯低级错误,自家产品没人能弄明白
ant-design的Select组件采用自定义后缀图标(suffixIcon属性)时,点击该自定义图标没有反应,不会展示下拉菜单的问题
Hey, I had another fight with HR in the small group!
Example 041: Methods and variables of a class
Multithreading JUC Learning Chapter 1 Steps to Create Multithreading
Continuing to invest in product research and development, Dingdong Maicai wins in supply chain investment
idea中diagram使用
Engineering drawing review questions (with answers)
STM8S项目创建(STVD创建)---使用 COSMIC 创建 C 语言项目
Presto中broadcast join和partition join执行计划的处理过程
织梦内核电动伸缩门卷闸门门业公司网站模板 带手机版【站长亲测】
C program compilation and predefined detailed explanation
C程序编译和预定义详解
大佬们,读取mysql300万单表要很长时间,有什么参数可以优惠,或者有什么办法可以快点
Oracle迁移到瀚高之后,空值问题处理
Example 037: Sorting
持续投入商品研发,叮咚买菜赢在了供应链投入上
简单排序(暑假每日一题 14)
持续投入商品研发,叮咚买菜赢在了供应链投入上