Builder pattern application scenarios :
It is mainly used to build some complex objects , Here's a complex object like : When building buildings , We need to lay the foundation first , Building framework , And then cover it from bottom to top . Usually , In the construction of such complex structures , It's hard to do it all at once . We need to build parts of this object first , And then put them together in stages . So now there's the builder pattern : The builder pattern is made up of Builder( builder ) and Director( commander ) form . Please see the following UML chart :
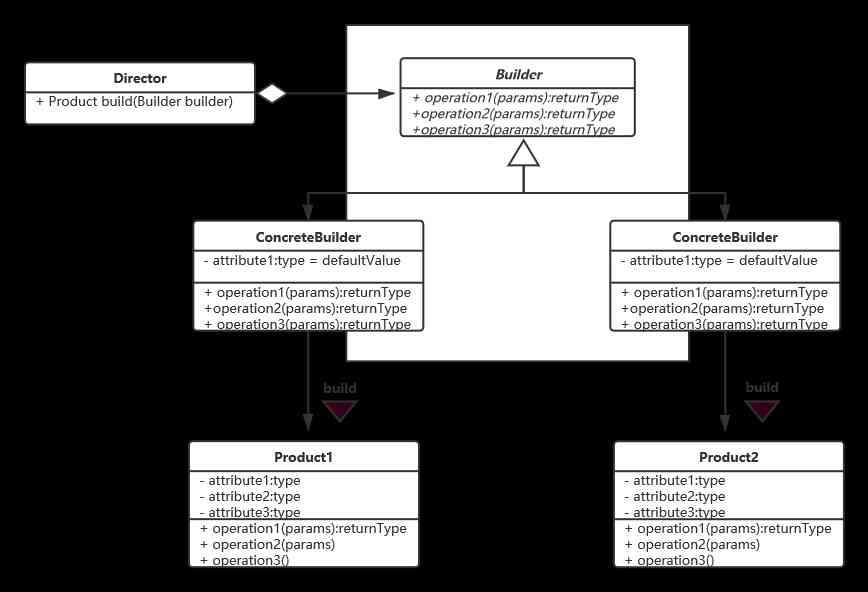
Director class
package com.yuan.builder.demo1;
// command : The core , Responsible for directing the construction of a project , How the project builds , It's up to it public class Director {
// Instruct the workers to give the order of execution public Product build(Builder builder) { builder.buildA(); builder.buildC(); builder.buildD(); builder.buildB(); return builder.getProduct(); }
}
Builder class
package com.yuan.builder.demo1; // The abstract builder public abstract class Builder {
abstract void buildA();// The foundation abstract void buildB();// Steel Engineering abstract void buildC();// Laying wires abstract void buildD();// Paint
// to be finished : Got a specific product abstract Product getProduct(); }
Worker class
package com.yuan.builder.demo1;
/** * The concrete builder : Worker */ public class Worker extends Builder { private Product product;
public Worker() { this.product = new Product(); }
@Override void buildA() { product.setBuildA(" The foundation "); System.out.println(" The foundation "); }
@Override void buildB() { product.setBuildB(" Steel Engineering "); System.out.println(" Steel Engineering "); }
@Override void buildC() { product.setBuildC(" Laying wires "); System.out.println(" Laying wires "); }
@Override void buildD() { product.setBuildD(" Paint "); System.out.println(" Paint "); }
@Override Product getProduct() { return product; } }
Product class
package com.yuan.builder.demo1; // product : house public class Product {
private String buildA; private String buildB; private String buildC; private String buildD;
public String getBuildA() { return buildA; }
public void setBuildA(String buildA) { this.buildA = buildA; }
public String getBuildB() { return buildB; }
public void setBuildB(String buildB) { this.buildB = buildB; }
public String getBuildC() { return buildC; }
public void setBuildC(String buildC) { this.buildC = buildC; }
public String getBuildD() { return buildD; }
public void setBuildD(String buildD) { this.buildD = buildD; }
@Override public String toString() { return "Product{" + "buildA='" + buildA + '\'' + ", buildB='" + buildB + '\'' + ", buildC='" + buildC + '\'' + ", buildD='" + buildD + '\'' + '}'; } }
Test class
package com.yuan.builder.demo1;
public class Test { public static void main(String[] args) { // command Director director = new Director(); // Direct specific workers to complete Product product = director.build(new Worker()); System.out.println(product.toString()); } }
|
Development train of thought :
Who knows what : In object oriented programming ,“ Who knows what ” It's very important . in other words , We need to pay attention to which class can be applied to which method and which method to use when programming .
Things that can be decided and things that can't be decided in design :Builder The definition of abstract methods in a class !!!