当前位置:网站首页>C language helps you understand pointers from multiple perspectives (1. Character pointers 2. Array pointers and pointer arrays, array parameter passing and pointer parameter passing 3. Function point
C language helps you understand pointers from multiple perspectives (1. Character pointers 2. Array pointers and pointer arrays, array parameter passing and pointer parameter passing 3. Function point
2022-07-07 20:30:00 【Zhu C】
Catalog
2. Array pointer and pointer array :
5. A pointer to an array of function pointers :
1. Character pointer :
Character pointer , seeing the name of a thing one thinks of its function , Pointer to character data , It is also the simplest kind of pointer introduced in this article , But there are a few fallible points , So take this opportunity to explain in detail . The following is the simplest use of character pointers
int main()
{
char ch = 'w';
char *pc = &ch;
*pc = 'w';
return 0; }
besides , We often encounter situations where character pointers point to strings :
int main()
{
const char* pstr = "hello world.";
printf("%s\n", pstr);
return 0; }
Here is to put a string into pstr Is it in the pointer variable ?
Obviously not , But why can I print this string ?
as a result of , The character pointer stores the address of the first element of the string , then printf Function %s Look down with the address of the first element , Print out the whole string . therefore , in fact , The character pointer only stores the address of the first element of the string .
Now let's give a topic , Consolidate the most important points of character pointer :
#include<stdio.h>
int main()
{
char str1[] = "hello world.";
char str2[] = "hello world.";
const char* str3 = "hello world.";//3 4 Constant string , Cannot be changed , In the read-only data area
const char* str4 = "hello world.";
if (str1 == str2)// The array name is the first element address ,
//1 and 2 Are two separate arrays , So the first address is different
printf("str1 == str2\n");
else
printf("str1 != str2\n");
if (str3 == str4)// explain str3 and str4 Point to the same string , All are a The address of
printf("str3 == str4\n");
else
printf("str3 != str4\n");
return 0;
}
2. Array pointer and pointer array :
Understanding pointers in depth can avoid distinguishing pointer arrays from array pointers and learning to apply .
First of all, it is clear that the array pointer is The pointer ! Pointer array is used to store pointers Array !
// Pointer array :
int *p1[10];
// Array pointer :
int (*p2)[10];
Explanation of array pointer :
#include <stdio.h>
int main()
{
int arr[10] = {0};
printf("%p\n", arr);
printf("%p\n", &arr);
return 0; }
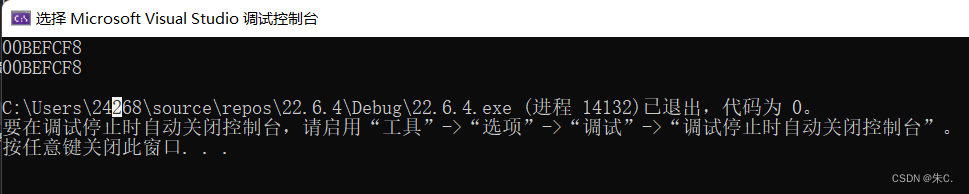
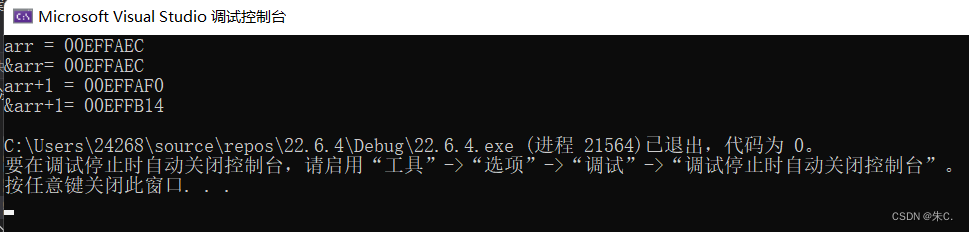
Here we find ,&arr Actually arr The address of the entire array ,+1 Then skip the length of the entire array .
#include <stdio.h>
int main()
{
int arr[10] = {1,2,3,4,5,6,7,8,9,0};
int (*p)[10] = &arr;// Put the array arr To an array pointer variable p
// But we seldom write code like this
return 0; }
#include <stdio.h>
void print_arr1(int arr[3][5], int row, int col) {
int i = 0;
for(i=0; i<row; i++)
{
for(j=0; j<col; j++)
{
printf("%d ", arr[i][j]);
}
printf("\n");
}
}
void print_arr2(int (*arr)[5], int row, int col) {
int i = 0;
for(i=0; i<row; i++)
{
for(j=0; j<col; j++)
{
printf("%d ", arr[i][j]);
}
printf("\n");
}
}
int main()
{
int arr[3][5] = {1,2,3,4,5,6,7,8,9,10};
print_arr1(arr, 3, 5);
// Array name arr, Represents the address of the first element
// But the first element of a two-dimensional array is the first row of a two-dimensional array
// So the message here is arr, It's actually equivalent to the address on the first line , Is the address of a one-dimensional array
// You can use an array pointer to receive
print_arr2(arr, 3, 5);
return 0; }
The array pointer is used in the parameter passing of the second code , Please pay attention to the difference between the two
3. A function pointer :
For different functions , In fact, we can also point to the corresponding function with a pointer , And it is used to call the function by dereferencing the function pointer , Here is a simple example for understanding :
int Add(int x,int y)
{
return x + y;
}
int main()
{
int arr[5] = { 0 };
int(*p)[5] = &arr;// Array pointer
//& Function name -- What you get is the address of the function ?
printf("%p\n", &Add);// Functions have addresses
printf("%p\n", Add);// There is no difference between the two ,& Function name and function name are the address of the function
int (*pf)(int, int) = &Add;// Similar to array pointers
int ret=(*pf)(2, 3);//Add(2,3)
int ret = pf(2, 3);// It's fine too
printf("%d\n", ret);
return 0;
}
We compare array pointers , After the array pointer [ ] Replaced by the function used ( ) It is roughly the rudiment of function pointer , Form like
int(*pf)(int x,int y) there * Express pf Is a pointer ,( ) Indicates a pointer to a function .
#include <stdio.h>
void test()
{
printf("hehe\n");
}
int main()
{
printf("%p\n", test);
printf("%p\n", &test);
return 0; }
Through this function and output, we found that in fact, functions also have addresses , This also proves the rationality of the existence of function pointers . A preliminary understanding of function pointers lays the foundation for the three categories we will introduce below .
4. Function pointer array :
Function pointer array , Through our understanding above , Is an array of function pointers , Then how to write its form ? Now we give :int (*parr1[10])();
In fact, it is not difficult for us to figure out , Put an array on the basis of the function pointer , So this array is used to store function pointers . Next, we give an example of implementing a computer to further explain the function pointer array ( The calculator is relatively simple , An example intended to implement a function pointer array ):
#include <stdio.h>
int add(int a, int b) {
return a + b;
}
int sub(int a, int b) {
return a - b;
}
int mul(int a, int b) {
return a * b;
}
int div(int a, int b) {
return a / b;
}
int main()
{
int x, y;
int input = 1;
int ret = 0;
int(*p[5])(int x, int y) = { 0, add, sub, mul, div }; // Transfer table
while (input)
{
printf("*************************\n");
printf(" 1:add 2:sub \n");
printf(" 3:mul 4:div \n");
printf("*************************\n");
printf(" Please select :");
scanf("%d", &input);
if ((input <= 4 && input >= 1))
{
printf(" Enter the operands :");
scanf("%d %d", &x, &y);
ret = (*p[input])(x, y);
}
else
printf(" Incorrect input \n");
printf("ret = %d\n", ret);
}
return 0;
}
Note that the function pointer array is used in the middle of the transfer table , It can point to the corresponding function implementation .
5. A pointer to an array of function pointers :
With the above, we understand , We can try to write out the form of pointers to the array of function pointers ,
Let's dissect :
The pointer to the array of function pointers is a The pointer
void test(const char* str) {
printf("%s\n", str);
}
int main()
{
// A function pointer pfun
void (*pfun)(const char*) = test;
// An array of function pointers pfunArr
void (*pfunArr[5])(const char* str);
pfunArr[0] = test;
// Pointer to function array pfunArr The pointer to ppfunArr
void (*(*ppfunArr)[5])(const char*) = &pfunArr;
return 0; }
Here we have a brief understanding , Finally, we give the implementation process of the callback function , This is the most important example , It is also a classic with technical content in pointer .
6. Callback function :
Definition :
We borrow qsort Function , And do it yourself qsort Function to call back
// Bubble sort can only sort integer data
//void bubble_sort(int arr[],int sz)
//{
// int i = 0;
// int j = 0;
//for(i=0;i<sz-1;i++)
//{int flag = 1;// Suppose the array is well ordered
// for (j = 0;j<sz-i-1;j++)
// {
//
// if (arr[j] > arr[j + 1])
// {
// int tmp = arr[j];
// arr[j] = arr[j + 1];
// arr[j + 1] = tmp;
// flag = 0;//
// }
// }
// if(flag==1)
// {
// break;
// }
//}
//}
//qsort Library function A sort function implemented using the idea of quick sort
// You can sort any type of data
//
//
//void qsort(void* base,// The starting position of the data you want to sort
//size_t num,// The number of data elements to be sorted
//size_t width, // The size of the data elements to be sorted
//int(* cmp)(const void* e1, const void* e2)// A function pointer , It's a comparison function
//);
// Compare two shaping elements
//e1 Point to an integer
//e2 Point to an integer
int cmp_int(const void* e1, const void* e2)
//{
// if (*(int*)e1 > *(int*)e2)// Cast
// return 1;
// else if (*(int*)e1 == *(int*)e2)
// return 0;
// else
// return -1;
//}
//
{return *(int*)e1 - *(int*)e2; }// Cast
void Swap(char* buf1, char* buf2, int width)
{
int i = 0;
for (i = 0; i < width; i++)
{
char tmp = *buf1;
*buf1 = *buf2;
*buf2 = tmp;
buf1++;
buf2++;
}
}
//void* It cannot be dereferenced directly
//void Is a pointer without a specific type , Can accept any type of pointer
// No specific type , So you can't dereference , Also can not +- Integers
void bubble_sort(void* base, int sz, int width, int(*cmp)(const void* e1, const void* e2))
{
int i = 0;
// Number of trips
for (i = 0; i < sz - 1; i++)
{
int flag = 1;// Suppose the array is well ordered
// A bubble sorting process
int j = 0;
for (j = 0; j < sz - 1 - i; j++)
{
if (cmp((char*)base + j * width, (char*)base + (j + 1) * width) > 0)
{
// In exchange for
Swap((char*)base + j * width, (char*)base + (j + 1) * width, width);
flag = 0;
}
}
if (flag == 1)
{
break;
}
}
}
struct Stu
{
char name[20];
int age;
};
int cmp_stu_by_name(const void* e1,const void* e2)
{
return strcmp(((struct Stu*)e1)->name, ((struct Stu*)e2)->name);
}
void test1()
{
int arr[] = { 9,8,7,6,5,4,3,2,1,0 };
// Arrange the array in ascending order
int sz = sizeof(arr) / sizeof(arr[0]);
//bubble_sort(arr, sz);
qsort(arr, sz, sizeof(arr[0]), cmp_int);// Default ascending
int i = 0;
for (i = 0; i < sz; i++)
{
printf("%d ", arr[i]);
}
return 0;
}
void test2()
{// Test use qsort To sort structure data
struct Stu s[] = { {"zs",15 }, { "ls",30 }, { "ww",25 } };
int sz = sizeof(s) / sizeof(s[0]);
qsort(s, sz, sizeof(s[0]),cmp_stu_by_name);
}
void test3()
{
int arr[] = { 9,8,7,6,5,4,3,2,1,0 };
// Arrange the array in ascending order
int sz = sizeof(arr) / sizeof(arr[0]);
//bubble_sort(arr, sz);
bubble_sort(arr, sz, sizeof(arr[0]), cmp_int);// Default ascending
int i = 0;
for (i = 0; i < sz; i++)
{
printf("%d ", arr[i]);
}
return 0;
}
void test4()
{
// Test use qsort To sort structural data
struct Stu s[] = { {"zhangsan", 15}, {"lisi", 30}, {"wangwu", 25} };
int sz = sizeof(s) / sizeof(s[0]);
bubble_sort(s, sz, sizeof(s[0]), cmp_stu_by_name);
//bubble_sort(s, sz, sizeof(s[0]), cmp_stu_by_age);
}
int main()
{
//test1();
//test2();
test3();
test4();
}
Here we give detailed code comments in the code . Here we are mainly in bubble_sort Callback in function cmp_XX Corresponding function , Please also understand carefully .
The above is the content of the advanced pointer , In the future, we will use this knowledge many times in the data structure . Hope to help you !
边栏推荐
- 智能软件分析平台Embold
- 备份 TiDB 集群到持久卷
- 如何挑选基金产品?2022年7月份适合买什么基金?
- Implement secondary index with Gaussian redis
- 论文解读(ValidUtil)《Rethinking the Setting of Semi-supervised Learning on Graphs》
- FTP steps for downloading files from Huawei CE switches
- [résolution] le paquet « xxxx» n'est pas dans goroot
- 微服务远程Debug,Nocalhost + Rainbond微服务开发第二弹
- Tensorflow2.x下如何运行1.x的代码
- 【解决】package ‘xxxx‘ is not in GOROOT
猜你喜欢
Cantata9.0 | 全 新 功 能
程序猿赚的那点钱算个P啊!
目标:不排斥 yaml 语法。争取快速上手
Micro service remote debug, nocalhost + rainbow micro service development second bullet
ERROR: 1064 (42000): You have an error in your SQL syntax; check the manual that corresponds to your
The latest version of codesonar has improved functional security and supports Misra, c++ parsing and visualization
Network principle (1) - overview of basic principles
嵌入式系统真正安全了吗?[ OneSpin如何为开发团队全面解决IC完整性问题 ]
智能软件分析平台Embold
VMWare中虚拟机网络配置
随机推荐
如何满足医疗设备对安全性和保密性的双重需求?
Details of C language integer and floating-point data storage in memory (including details of original code, inverse code, complement, size end storage, etc.)
ERROR: 1064 (42000): You have an error in your SQL syntax; check the manual that corresponds to your
【网络原理的概念】
TS quick start - Generic
H3C s7000/s7500e/10500 series post stack BFD detection configuration method
实战:sqlserver 2008 扩展事件-XML转换为标准的table格式[通俗易懂]
使用 BR 备份 TiDB 集群数据到 Azure Blob Storage
【解决】package ‘xxxx‘ is not in GOROOT
When easygbs cascades, how to solve the streaming failure and screen jam caused by the restart of the superior platform?
VMWare中虚拟机网络配置
数值法求解最优控制问题(〇)——定义
深度学习模型压缩与加速技术(七):混合方式
【C语言】指针进阶---指针你真的学懂了吗?
Phoenix JDBC
Airiot helps the urban pipe gallery project, and smart IOT guards the lifeline of the city
[résolution] le paquet « xxxx» n'est pas dans goroot
使用高斯Redis实现二级索引
4G设备接入EasyGBS平台出现流量消耗异常,是什么原因?
恶魔奶爸 B1 听力最后壁垒,一鼓作气突破