当前位置:网站首页>Dynamic memory management
Dynamic memory management
2022-07-04 09:47:00 【skeet follower】
Catalog
1. Why dynamic memory management exists
2. The introduction of dynamic memory function
3. Common dynamic memory errors
4.2 The characteristics of flexible arrays
4.3 The use of flexible arrays
4.4 Advantages of flexible arrays
1. Why dynamic memory management exists
Of course, we have mastered the way of memory development :
int a=10;// local variable - Four spaces are opened up in the stack space
int g_a=10;// Global variables - Static zone
But there are two characteristics of the way to open up space :
struct stu{
char name[20];
int age;
}
int main(){
int n=0;
scanf("%d",&n);
struct stu arr[n];
return 0;
}
Yes C99 This standard is introduced in , But many compilers do not support this standard , So it will report an error , Therefore, this method is not feasible .
2. The introduction of dynamic memory function
2.1malloc and free
Dynamic memory development function code example :
void* malloc (size_t size);
This function requests a piece of memory Continuously available Space , And return the pointer to this space .
- If the development is successful , Then return a pointer to open a good space .
- If the development fails , Returns a NULL The pointer , therefore malloc The return value of must be checked .
- The type of return value is void* , therefore malloc Function doesn't know the type of open space , Use yourself to decide when using .
void free (void* ptr);
free Function is used to release memory opened dynamically .
- If parameters ptr The pointed space is not opened dynamically , that free The behavior of a function is undefined .
- If parameters ptr yes NULL The pointer , Then the function does nothing .
#include<stdio.h>
#include<stdlib.h>
int main()
{
// Apply to memory 10 An integer space
int* p = (int*)malloc(10 * sizeof(int));//int* Force type to integer
if (p == NULL) {
// Failed to open up space , The reason for the printing error
printf("%s\n", strerror(errno));
}
else {
// Normal use space
int i = 0;
for (i = 0; i < 10; i++) {
*(p + i) = i;
}
for (i = 0; i < 10; i++) {
printf("%d", *(p + i));
}
}
// When dynamic memory space is not in use
// It should be returned to the operating system
free(p);
p = NULL;
return 0;
}
2.2calloc
calloc Function dynamic memory allocation . The prototype is as follows :
void* calloc (size_t num, size_t size);
- The function is for num Size is size The elements of open up a space , And initialize each byte of the space to 0.
- And functions malloc The only difference is calloc Initializes each byte of the requested space to full before returning the address 0. The code is as follows :
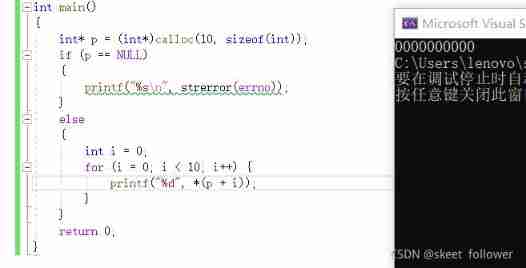
2.3realloc
- realloc Functions make dynamic memory management more flexible .
- Sometimes we find that the application space is too small in the past , Sometimes we think the application space is too large , That's for reasonable time memory , We will make flexible adjustments to the size of memory . that realloc Function can be used to adjust the dynamic memory size . The function prototype as follows :void* realloc (void* ptr, size_t size);
- ptr Is the memory address to be adjusted ,size New size after adjustment , The return value is the starting memory position after adjustment .
- This function adjusts the size of the original memory space , The original data in memory will also be moved to new Space .
#include<stdio.h>
#include<stdlib.h>
int main()
{
// Apply to memory 10 An integer space
int* p = (int*)malloc(20);//int* Force type to integer
if (p == NULL) {
// Failed to open up space , The reason for the printing error
printf("%s\n", strerror(errno));
}
else {
// Normal use space
int i = 0;
for (i = 0; i < 10; i++) {
*(p + i) = i;
}
}
int* ptr = realloc(p, 40);
if (ptr != NULL) {
p = ptr;
int i = 0;
for (i = 5; i < 10; i++) {
*(p + i) = i;
}
for (i = 0; i < 10; i++) {
printf("%d", *(p + i));
}
}
free(p);
p = NULL;
return 0;
}
3. Common dynamic memory errors
- Yes NULL Dereference operation of pointer
#include<stdio.h>
#include<stdlib.h>
int main()
{
int* p = (int*)malloc(40);
int i = 0;
for (i = 0; i < 10; i++)
{
*(p + i) = i;
}
free(p);
p = NULL;
return 0;
}
In case malloc Failed to open up space , be p Will be assigned a null pointer , therefore malloc When using, you must judge whether the return value is a null pointer .
- Cross border access to dynamic open space
#include<stdio.h>
#include<stdlib.h>
int main()
{
int* p = (int*)malloc(5 * sizeof(int));
if (p == NULL) {
return 0;
}
else {
int i = 0;
for (i = 0; i < 10; i++) {
*(p + i) = i;
}
}
free(p);
p = NULL;
return 0;
}
malloc Only opened up 5 An integer element , and for Loop access 10 Caused cross-border visits , Program crash .
- Use of non dynamic memory free Release
#include<stdio.h>
#include<stdlib.h>
int main()
{
int a = 10;
int* p = &a;
*p = 20;
free(p);
return 0;
}
- Use free Release a piece of dynamic memory
#include<stdio.h>
#include<stdlib.h>
int main()
{
int* p = (int*)malloc(40);
if (p == NULL) {
return 0;
}
else {
int i = 0;
for (i = 0; i < 10; i++) {
*p++ = i;
}
}
free(p);
p = NULL;
return 0;
}
p Changes have taken place in this process , It is not our complete development space , If you want to release, you can only release it from the starting position of the opening , So wrong .
- Multiple releases of the same dynamic memory
void test()
{
int *p = (int *)malloc(100);
free(p);
free(p);// Repeat release
}
- Dynamic memory forget to release ( Memory leak )
void test()
{
int *p = (int *)malloc(100);
if(NULL != p)
{
*p = 20;
}
}
int main()
{
test();
while(1);
}
No space is released after use , Will continue to consume memory , It may cause the server to crash .
4. Flexible array
4.1 Concept of flexible array
Maybe you've never heard of Flexible array (flflexible array) The concept , But it does exist . C99 in , The most in the structureThe latter element is allowed to be an array of unknown size , This is called 『 Flexible array 』 member
The code is as follows :
typedef struct st_type
{
int i;
int a[];// Flexible array members
}type_a;
4.2 The characteristics of flexible arrays
- A flexible array member in a structure must be preceded by at least one other member .
- sizeof The size of the structure returned does not include the memory of the flexible array .
- Structures that contain flexible array members use malloc () Function to dynamically allocate memory , And the allocated memory should be larger than the size of the structure , Adapt to Expected size of the flexible array .
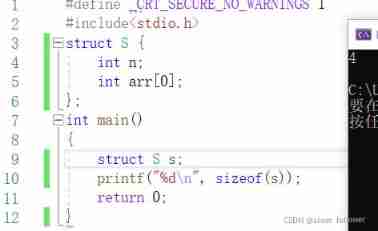
4.3 The use of flexible arrays
Code 1:
#include<stdio.h>
struct S {
int n;
int arr[0];
};
int main()
{
struct S* s = (struct S*)malloc(sizeof(struct S) + 5 * sizeof(int));
int i = 0;
for (i = 0; i < 5; i++) {
s->arr[i] = i;
}
struct S* ps = realloc(s, 44);
if (ps!= NULL) {
s = ps;
for (i = 5; i < 10; i++) {
s->arr[i] = i;
}
}
for (i = 0; i < 10; i++) {
printf("%d", s->arr[i]);
}
free(s);
s = NULL;
return 0;
}
Code 2:
#include<stdio.h>
struct S {
int n;
int* arr;
};
int main()
{
struct S* ps = (struct S*)malloc(sizeof(struct S) + 5 * sizeof(int));
ps->arr = malloc(5 * sizeof(int));
int i = 0;
for (i = 0; i < 5; i++) {
ps->arr[i] = i;
}
for (i = 0; i < 5; i++) {
printf("%d\n", ps->arr[i]);
}
int* ptr = realloc(ps->arr, 10 * sizeof(int));
if (ptr!= NULL) {
ps->arr = ptr;
for (i = 5; i < 10; i++) {
ps->arr[i] = i;
}
}
for (i = 0; i < 10; i++) {
printf("%d ", ps->arr[i]);
}
free(ps->arr);
free(ps);
ps = NULL;
return 0;
}
4.4 Advantages of flexible arrays
By comparing the above code 1 And code 2, Code 1 The implementation of has two benefits :
1. Convenient memory release
2. Conducive to access speed
边栏推荐
- Golang Modules
- C # use smtpclient The sendasync method fails to send mail, and always returns canceled
- Golang defer
- Multilingual Wikipedia website source code development part II
- How web pages interact with applets
- Luogu deep foundation part 1 Introduction to language Chapter 4 loop structure programming (2022.02.14)
- C # use gdi+ to add text with center rotation (arbitrary angle)
- MySQL develops small mall management system
- MySQL transaction mvcc principle
- Are there any principal guaranteed financial products in 2022?
猜你喜欢
Daughter love in lunch box
Sort out the power node, Mr. Wang he's SSM integration steps
智慧路灯杆水库区安全监测应用
2022-2028 global seeder industry research and trend analysis report
Logstack configuration details -- elasticstack (elk) work notes 020
百度研发三面惨遭滑铁卢:面试官一套组合拳让我当场懵逼
165 webmaster online toolbox website source code / hare online tool system v2.2.7 Chinese version
Summary of small program performance optimization practice
C语言指针经典面试题——第一弹
xxl-job惊艳的设计,怎能叫人不爱
随机推荐
Go context basic introduction
PHP student achievement management system, the database uses mysql, including source code and database SQL files, with the login management function of students and teachers
2022-2028 global strain gauge pressure sensor industry research and trend analysis report
Hands on deep learning (45) -- bundle search
pcl::fromROSMsg报警告Failed to find match for field ‘intensity‘.
查看CSDN个人资源下载明细
Daughter love: frequency spectrum analysis of a piece of music
The child container margin top acts on the parent container
SQL replying to comments
About the for range traversal operation in channel in golang
Kubernetes CNI 插件之Fabric
Global and Chinese market of sampler 2022-2028: Research Report on technology, participants, trends, market size and share
Intelligent gateway helps improve industrial data acquisition and utilization
Ultimate bug finding method - two points
H5 audio tag custom style modification and adding playback control events
MATLAB小技巧(25)竞争神经网络与SOM神经网络
Hands on deep learning (35) -- text preprocessing (NLP)
Modules golang
Problems encountered by scan, scanf and scanln in golang
Machine learning -- neural network (IV): BP neural network