当前位置:网站首页>Web APIs DOM node
Web APIs DOM node
2022-07-05 05:20:00 【Dark horse programmer official】
Notes updated in the early stage : Web API Basic cognition / obtain DOM Elements / Set up / modify DOM Element content and element attributes / Timer - Intermittent function / The basis of the event / Higher order function / Environment object / Comprehensive case -Tab Bar Toggle
One 、DOM node
DOM Every content in the tree is called a node
1.1 Node type
- Element nodes ( Focus on the element node Can better let us clarify the relationship between tag elements )
All the labels such as body、 div
html Root node
- Attribute node
All attributes such as href
- Text node
All the text
- other
1.2 Find node
Close the QR code case :
Click the close button , The box with QR code is closed , And get erweima The box
reflection :
- close button and erweima What is the relationship ?
- Father and son
- therefore , We can do this :
- Click the close button , Just close it, Dad , You don't need to get erweima Element
1.2.1 Node relationship :
- Parent node
- Child node
- Brother node
1.2.2 Parent node lookup :
- parentNode attribute
- Return the nearest parent node Not found. Return is null
Code :
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<div class="father">
<div class="son"> son </div>
</div>
<script>
let son = document.querySelector('.son')
// Look for Dad
// console.log(son.parentNode)
son.parentNode.style.display = 'none'
</script>
</body>
</html>
1.2.3 Close the QR code case
Code :
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
<style>
.erweima {
width: 149px;
height: 152px;
border: 1px solid #000;
background: url(./images/456.png) no-repeat;
position: relative;
}
.close {
position: absolute;
right: -52px;
top: -1px;
width: 50px;
height: 50px;
background: url(./images/bgs.png) no-repeat -159px -102px;
cursor: pointer;
border: 1px solid #000;
}
</style>
</head>
<body>
<div class="erweima">
<span class="close"></span>
</div>
<div class="erweima">
<span class="close"></span>
</div>
<div class="erweima">
<span class="close"></span>
</div>
<div class="erweima">
<span class="close"></span>
</div>
<div class="erweima">
<span class="close"></span>
</div>
<script>
// 1. Get elements close button
let close_btn = document.querySelectorAll('.close')
// 2. Bind multiple click events to close
for (let i = 0; i < close_btn.length; i++) {
close_btn[i].addEventListener('click', function () {
// 3. Close the current QR code Click who , Just close whose father
this.parentNode.style.visibility = 'hidden'
})
}
</script>
</body>
</html>
1.2.4 Find child nodes :
childNodes : Get all the child nodes 、 Include text nodes ( Space 、 Line break )、 Comment nodes, etc
children ( a key )
- Only get all element nodes
- What is returned is a pseudo array
Code :
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<button> Click on </button>
<ul>
<li> I'm a kid </li>
<li> I'm a kid </li>
<li> I'm a kid </li>
<li> I'm a kid </li>
<li> I'm a kid </li>
<li> I'm a kid </li>
</ul>
<script>
let btn = document.querySelector('button')
let ul = document.querySelector('ul')
btn.addEventListener('click', function () {
// console.log(ul.children)
for (let i = 0; i < ul.children.length; i++) {
ul.children[i].style.color = 'red'
}
})
ul.children[0].style.color = 'green'
// console.log(ul.childNodes)
</script>
</body>
</html>
1.2.5 Brotherhood search :
1. Next sibling node
* nextElementSibling attribute
2. Last sibling node
* previousElementSibling attribute
Code :
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<button> Click on </button>
<ul>
<li> The first 1 individual </li>
<li class="two"> The first 2 individual </li>
<li> The first 3 individual </li>
<li> The first 4 individual </li>
</ul>
<script>
let btn = document.querySelector('button')
let two = document.querySelector('.two')
btn.addEventListener('click', function () {
// two.style.color = 'red'
two.nextElementSibling.style.color = 'red'
two.previousElementSibling.style.color = 'red'
})
</script>
</body>
</html>
1.3 Add node
such as , Click the Publish button , You can add a message
- In general , We add new nodes , Do the following :
- Create a new node
- Put the created new node into the specified element
Code :
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<ul>
<li> I'm Mao </li>
<li> I'm twenty cents </li>
</ul>
<script>
// 20 Fen ul.children[1]
// 1. Create a new label node
// let div = document.createElement('div')
// div.className = 'current'
let ul = document.querySelector('ul')
let li = document.createElement('li')
li.innerHTML = ' I am a xiao ming'
// 2. Add nodes Parent element .appendChild( Subelement ) Back up
// ul.appendChild(li)
// 3. Add nodes Parent element .insertBefore( Subelement , Put it in front of that element )
ul.insertBefore(li, ul.children[0])
</script>
</body>
</html>
1.3.1 Create nodes
- That is to create a new web page element , Then add it to the web page , Generally, create nodes first , Then insert the node
- Create element node method :
1.3.2. Add nodes
- To see in the interface , You have to insert it into a parent element
- Insert into the last child element of the parent element :
Learn online case rendering demand : Render the page according to the data
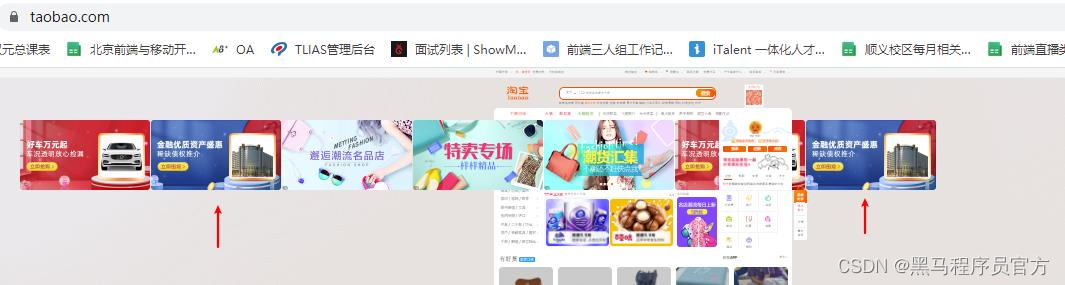
- Copy an existing node
- Put the copied node into the specified element
index.html Code :
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title> Learn to drive online home page </title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<!-- 4. box The core content area begins -->
<div class="box w">
<div class="box-hd">
<h3> Excellent recommendation </h3>
<a href="#"> Check all </a>
</div>
<div class="box-bd">
<ul class="clearfix">
<!-- <li>
<img src="./images/course01.png" alt="">
<h4>
Think PHP 5.0 Blog system actual combat project drill
</h4>
<div class="info">
<span> senior </span> • <span> 1125</span> People are learning
</div>
</li> -->
</ul>
</div>
</div>
<script>
let data = [
{
src: 'images/course01.png',
title: 'Think PHP 5.0 Blog system actual combat project drill ',
num: 1125
},
{
src: 'images/course02.png',
title: 'Android Network dynamic picture loading practice ',
num: 357
},
{
src: 'images/course03.png',
title: 'Angular2 Large front-end mall actual combat project drill ',
num: 22250
},
{
src: 'images/course04.png',
title: 'Android APP Actual combat project drill ',
num: 389
},
{
src: 'images/course05.png',
title: 'UGUI Source code depth analysis case ',
num: 124
},
{
src: 'images/course06.png',
title: 'Kami2 Home page interface switching effect actual combat drill ',
num: 432
},
{
src: 'images/course07.png',
title: 'UNITY From getting started to mastering practical cases ',
num: 888
},
{
src: 'images/course08.png',
title: ' I will change , And you? ?',
num: 590
},
{
src: 'images/course08.png',
title: ' I will change , And you? ?',
num: 590
}
]
let ul = document.querySelector('ul')
// 1. According to the number of data , Decide this little li The number of
for (let i = 0; i < data.length; i++) {
// 2. Create a small li
let li = document.createElement('li')
// console.log(li)
// 4. Prepare the content first , Additional
li.innerHTML = `
<img src=${data[i].src} alt="">
<h4>
${data[i].title}
</h4>
<div class="info">
<span> senior </span> • <span> ${data[i].num}</span> People are learning
</div>
`
// 3. Append to ul Parent element .appendChild( Subelement )
ul.appendChild(li)
}
</script>
</body>
</html>
css Code :
* {
margin: 0;
padding: 0;
}
.w {
width: 1200px;
margin: auto;
}
body {
background-color: #f3f5f7;
}
li {
list-style: none;
}
a {
text-decoration: none;
}
.clearfix:before,.clearfix:after {
content:"";
display:table;
}
.clearfix:after {
clear:both;
}
.clearfix {
*zoom:1;
}
.header {
height: 42px;
/* background-color: pink; */
/* Note that this place will be stacked w Inside margin */
margin: 30px auto;
}
.logo {
float: left;
width: 198px;
height: 42px;
}
.nav {
float: left;
margin-left: 60px;
}
.nav ul li {
float: left;
margin: 0 15px;
}
.nav ul li a {
display: block;
height: 42px;
padding: 0 10px;
line-height: 42px;
font-size: 18px;
color: #050505;
}
.nav ul li a:hover {
border-bottom: 2px solid #00a4ff;
color: #00a4ff;
}
/* search Search module */
.search {
float: left;
width: 412px;
height: 42px;
margin-left: 70px;
}
.search input {
float: left;
width: 345px;
height: 40px;
border: 1px solid #00a4ff;
border-right: 0;
color: #bfbfbf;
font-size: 14px;
padding-left: 15px;
}
.search button {
float: left;
width: 50px;
height: 42px;
/* Button button By default, there is a border that needs to be removed manually */
border: 0;
background: url(images/btn.png);
}
.user {
float: right;
line-height: 42px;
margin-right: 30px;
font-size: 14px;
color: #666;
}
/* banner Area */
.banner {
height: 421px;
background-color: #1c036c;
}
.banner .w {
height: 421px;
background: url(images/banner2.png) no-repeat top center;
}
.subnav {
float: left;
width: 190px;
height: 421px;
background: rgba(0,0,0, 0.3);
}
.subnav ul li {
height: 45px;
line-height: 45px;
padding: 0 20px;
}
.subnav ul li a {
font-size: 14px;
color: #fff;
}
.subnav ul li a span {
float: right;
}
.subnav ul li a:hover {
color: #00a4ff;
}
.course {
float: right;
width: 230px;
height: 300px;
background-color: #fff;
/* Floating boxes don't have the problem of outer margin merging */
margin-top: 50px;
}
.course h2 {
height: 48px;
background-color: #9bceea;
text-align: center;
line-height: 48px;
font-size: 18px;
color: #fff;
}
.bd {
padding: 0 20px;
}
.bd ul li {
padding: 14px 0;
border-bottom: 1px solid #ccc;
}
.bd ul li h4 {
font-size: 16px;
color: #4e4e4e;
}
.bd ul li p {
font-size: 12px;
color: #a5a5a5;
}
.bd .more {
display: block;
height: 38px;
border: 1px solid #00a4ff;
margin-top: 5px;
text-align: center;
line-height: 38px;
color: #00a4ff;
font-size: 16px;
font-weight: 700;
}
/* Boutique recommendation module */
.goods {
height: 60px;
background-color: #fff;
margin-top: 10px;
box-shadow: 0 2px 3px 3px rgba(0,0,0, 0.1);
/* Xinggao will inherit , Will inherit to 3 A child */
line-height: 60px;
}
.goods h3 {
float: left;
margin-left: 30px;
font-size: 16px;
color: #00a4ff;
}
.goods ul {
float: left;
margin-left: 30px;
}
.goods ul li {
float: left;
}
.goods ul li a {
padding: 0 30px;
font-size: 16px;
color: #050505;
border-left: 1px solid #ccc;
}
.mod {
float: right;
margin-right: 30px;
font-size: 14px;
color: #00a4ff;
}
.box {
margin-top: 30px;
}
.box-hd {
height: 45px;
}
.box-hd h3 {
float: left;
font-size: 20px;
color: #494949;
}
.box-hd a {
float: right;
font-size: 12px;
color: #a5a5a5;
margin-top: 10px;
margin-right: 30px;
}
/* hold li Father ul The modification is wide enough to fit a line 5 A box won't wrap */
.box-bd ul {
width: 1225px;
}
.box-bd ul li {
position: relative;
top: 0;
float: left;
width: 228px;
height: 270px;
background-color: #fff;
margin-right: 15px;
margin-bottom: 15px;
transition: all .3s;
}
.box-bd ul li:hover {
top: -8px;
box-shadow: 2px 2px 2px 2px rgba(0,0,0,.3);
}
.box-bd ul li img {
width: 100%;
}
.box-bd ul li h4 {
margin: 20px 20px 20px 25px;
font-size: 14px;
color: #050505;
font-weight: 400;
}
.box-bd .info {
margin: 0 20px 0 25px;
font-size: 12px;
color: #999;
}
.box-bd .info span {
color: #ff7c2d;
}
/* footer modular */
.footer {
height: 415px;
background-color: #fff;
}
.footer .w {
padding-top: 35px;
}
.copyright {
float: left;
}
.copyright p {
font-size: 12px;
color: #666;
margin: 20px 0 15px 0;
}
.copyright .app {
display: block;
width: 118px;
height: 33px;
border: 1px solid #00a4ff;
text-align: center;
line-height: 33px;
color: #00a4ff;
font-size: 16px;
}
.links {
float: right;
}
.links dl {
float: left;
margin-left: 100px;
}
.links dl dt {
font-size: 16px;
color: #333;
margin-bottom: 5px;
}
.links dl dd a {
color: #333;
font-size: 12px;
}
js Comprehensive case data code :
let data = [
{
src: 'images/course01.png',
title: 'Think PHP 5.0 Blog system actual combat project drill ',
num: 1125
},
{
src: 'images/course02.png',
title: 'Android Network dynamic picture loading practice ',
num: 357
},
{
src: 'images/course03.png',
title: 'Angular2 Large front-end mall actual combat project drill ',
num: 22250
},
{
src: 'images/course04.png',
title: 'Android APP Actual combat project drill ',
num: 389
},
{
src: 'images/course05.png',
title: 'UGUI Source code depth analysis case ',
num: 124
},
{
src: 'images/course06.png',
title: 'Kami2 Home page interface switching effect actual combat drill ',
num: 432
},
{
src: 'images/course07.png',
title: 'UNITY From getting started to mastering practical cases ',
num: 888
},
{
src: 'images/course08.png',
title: 'Cocos In depth study, you will not miss the actual battle ',
num: 590
},
]
1.3.3 Clone node

cloneNode Will clone an element that is the same as the original tag , Pass in Boolean values in parentheses
- if true, It means that the descendant nodes will be cloned together when cloning
- if false, It means that the descendant node is not included in the cloning
- The default is false
Code :
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<ul>
<li> I am content. 11111</li>
</ul>
<script>
let ul = document.querySelector('ul')
// If the bracket is empty, it defaults to false If it is false Do not clone descendant nodes
// If it is true Clone descendant nodes
let newUl = ul.cloneNode(true)
document.body.appendChild(newUl)
</script>
</body>
</html>
1.4 Delete node
- If a node is no longer needed in the page , You can delete it
- stay JavaScript Native DOM In operation , To delete an element, you must pass The parent element is deleted
- grammar
- notes :
If there is no parent-child relationship, the deletion is unsuccessful
Delete nodes and hide nodes (display:none) There is a difference : Hidden nodes still exist , But delete , From html Delete node
Code :
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<button> Click on </button>
<ul>
<li> I am content. 11111</li>
</ul>
<script>
// demand , Click button , Delete small li
let btn = document.querySelector('button')
let ul = document.querySelector('ul')
btn.addEventListener('click', function () {
// Deleted Syntax Parent element .removeChild( Subelement )
ul.removeChild(ul.children[0])
})
</script>
</body>
</html>
Dark horse front-end column has a lot of dry goods , Focus on relearning , It's convenient ~
2022 Front end learning roadmap : Course 、 Source code 、 note , Technology stack In addition, the circuit diagram is updated in real time ! Friends who need after-school materials , You can tell me directly .
边栏推荐
- What is the agile proportion of PMP Exam? Dispel doubts
- Magnifying glass effect
- [轉]: OSGI規範 深入淺出
- 2022年上半年国家教师资格证考试
- To be continued] [UE4 notes] L4 object editing
- [es practice] use the native realm security mode on es
- Three dimensional dice realize 3D cool rotation effect (with complete source code) (with animation code)
- 第六章 数据流建模—课后习题
- Animation
- 小程序直播+电商,想做新零售电商就用它吧!
猜你喜欢
随机推荐
[leetcode] integer inversion [7]
2022/7/1 learning summary
Three dimensional dice realize 3D cool rotation effect (with complete source code) (with animation code)
xftp7与xshell7下载(官网)
Embedded database development programming (V) -- DQL
Service fusing hystrix
《动手学深度学习》学习笔记
Unity enables mobile phone vibration
Programmers' experience of delivering takeout
软件测试 -- 0 序
[turn to] MySQL operation practice (III): table connection
cocos_ Lua loads the file generated by bmfont fnt
room数据库的使用
十年不用一次的JVM调用
A new micro ORM open source framework
Quick sort summary
Cocos2dx Lua registers the touch event and detects whether the click coordinates are within the specified area
[转]:Apache Felix Framework配置属性
Embedded database development programming (VI) -- C API
JVM call not used once in ten years