当前位置:网站首页>云原生应用开发之 gRPC 入门
云原生应用开发之 gRPC 入门
2022-07-07 23:48:00 【InfoQ】
什么是 gRPC
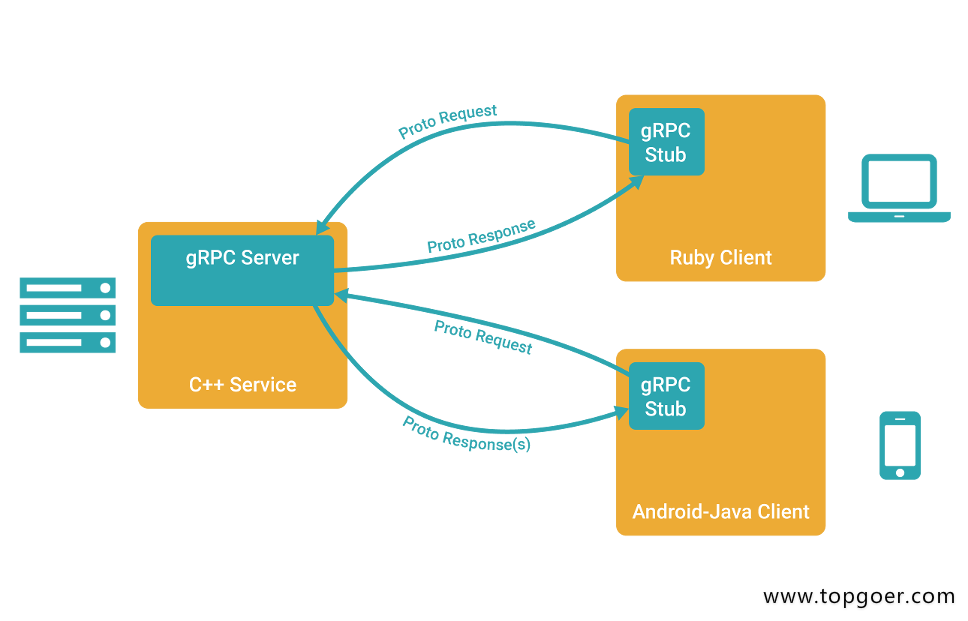
特点
- gRPC 是一个高性能、开源和通用的 RPC 框架,面向移动和 HTTP/2 设计,带来诸如双向流、流控、头部压缩、单 TCP 连接上的多复用请求等特。这些特性使得其在移动设备上表现更好,更省电和节省空间占用。
- 在 gRPC 里客户端应用可以像调用本地对象一样直接调用另一台不同的机器上服务端应用的方法,使得您能够更容易地创建分布式应用和服务。
- gRPC 默认使用 protocol buffers,这是 Google 开源的一套成熟的结构数据序列化机制,它的作用与 XML、json 类似,但它是二进制格式,性能好、效率高(缺点:可读性差)。
gRPC 和 REST 区别
- gRPC 使用 HTTP/2 协议,而 REST 使用 HTTP 1.1
- gRPC 使用协议缓冲区数据格式,而不是通常在 REST API 中使用的标准 JSON 数据格式
- 使用 gRPC,您可以根据需要利用 HTTP/2 功能,例如服务器端流式传输、客户端流式传输甚至双向流式传输。
Go 建立一个 gRPC 服务器
- 安装 golang 的proto工具包:
go get -u github.com/golang/protobuf/proto
- 在开始建立 gRPC 之前,确保已安装 Protocol Buffers v3:
go get -u github.com/golang/protobuf/protoc-gen-go
- 在 Go 中安装 gRPC:
go get google.golang.org/grpc
main
package main
import (
"log"
"net"
)
func main() {
lis, err := net.Listen("tcp", ":8000")
if err != nil {
log.Fatalf("Fail to listen: %v", err)
}
}
package main
import (
"log"
"net"
"google.golang.org/grpc"
)
func main() {
lis, err := net.Listen("tcp", ":8000")
if err != nil {
log.Fatalf("Fail to listen: %v", err)
}
grpcServer := grpc.NewServer()
if err := grpcServer.Serve(lis); err != nil {
log.Fatalf("Fail to serve: %v", err)
}
}
添加一些功能
client.proto
syntax = "proto3"; // 协议为proto3
package chat;
// 定义发送请求信息
message Message {
// 定义发送的参数
// 参数类型 参数名 标识号(不可重复)
string body = 1;
}
// 定义我们的服务(可定义多个服务,每个服务可定义多个接口)
service ChatService {
rpc SayHello(Message) returns (Message) {}
}
.proto
ChatService
SayHello
.proto
$ protoc --go_out=plugins=grpc:chat chat.proto
chat/chat.pb.go
server.go
package main
import (
"fmt"
"log"
"net"
"github.com/tutorialedge/go-grpc-beginners-tutorial/chat"
"google.golang.org/grpc"
)
func main() {
fmt.Println("Go gRPC Beginners Tutorial!")
lis, err := net.Listen("tcp", fmt.Sprintf(":%d", 9000))
if err != nil {
log.Fatalf("failed to listen: %v", err)
}
s := chat.Server{}
grpcServer := grpc.NewServer()
chat.RegisterChatServiceServer(grpcServer, &s)
if err := grpcServer.Serve(lis); err != nil {
log.Fatalf("failed to serve: %s", err)
}
}
package chat
import (
"log"
"golang.org/x/net/context"
)
type Server struct {
}
func (s *Server) SayHello(ctx context.Context, in *Message) (*Message, error) {
log.Printf("Receive message body from client: %s", in.Body)
return &Message{Body: "Hello From the Server!"}, nil
}
chat.proto
$ go run server.go
Go gRPC Beginners Tutorial!
localhost:8000
在 Go 中构建 gRPC 客户端
client.go
package main
import (
"log"
"golang.org/x/net/context"
"google.golang.org/grpc"
"github.com/tutorialedge/go-grpc-beginners-tutorial/chat"
)
func main() {
var conn *grpc.ClientConn
conn, err := grpc.Dial(":8000", grpc.WithInsecure())
if err != nil {
log.Fatalf("did not connect: %s", err)
}
defer conn.Close()
c := chat.NewChatServiceClient(conn)
response, err := c.SayHello(context.Background(), &chat.Message{Body: "Hello From Client!"})
if err != nil {
log.Fatalf("Error when calling SayHello: %s", err)
}
log.Printf("Response from server: %s", response.Body)
}
$ go run client.go
2022/07/07 23:23:01 Response from server: Hello From the Server!
安装问题
go get google.golang.org/grpc

- git clone https://github.com/grpc/grpc-go.git $GOPATH/src/google.golang.org/grpc
- git clone https://github.com/golang/net.git $GOPATH/src/golang.org/x/net
- git clone https://github.com/golang/text.git $GOPATH/src/golang.org/x/text
- go get -u github.com/golang/protobuf/{proto,protoc-gen-go}
- git clone https://github.com/google/go-genproto.git $GOPATH/src/google.golang.org/genproto
- cd $GOPATH/src/
- go install google.golang.org/grpc
总结
- Go gRPC Beginners Tutorial
- 《gRPC 与云原生应用开发》
边栏推荐
- Common effects of line chart
- Redis集群
- 2022 safety officer-b certificate examination question bank and safety officer-b certificate simulation test questions
- Leetcode exercise - Sword finger offer 36 Binary search tree and bidirectional linked list
- A little experience from reading "civilization, modernization, value investment and China"
- Anaconda3 download address Tsinghua University open source software mirror station
- Matlab code about cosine similarity
- Chapter improvement of clock -- multi-purpose signal modulation generation system based on ambient optical signal detection and custom signal rules
- QT -- package the program -- don't install qt- you can run it directly
- Frrouting BGP protocol learning
猜你喜欢
ArrayList源码深度剖析,从最基本的扩容原理,到魔幻的迭代器和fast-fail机制,你想要的这都有!!!
子矩阵的和
Solve the error: NPM warn config global ` --global`, `--local` are deprecated Use `--location=global` instead.
2021 tea master (primary) examination materials and tea master (primary) simulation test questions
qt--将程序打包--不要安装qt-可以直接运行
4. Strategic Learning
从cmath文件看名字是怎样被添加到命名空间std中的
碳刷滑环在发电机中的作用
About snake equation (2)
2021-03-06 - play with the application of reflection in the framework
随机推荐
[loss function] entropy / relative entropy / cross entropy
5. Discrete control and continuous control
Redis 主从复制
ROS problems (topic types do not match, topic datatype/md5sum not match, MSG XXX have changed. rerun cmake)
用户之声 | 对于GBase 8a数据库学习的感悟
2022 operation certificate examination for main principals of hazardous chemical business units and main principals of hazardous chemical business units
2022 high voltage electrician examination skills and high voltage electrician reexamination examination
子矩阵的和
Matlab code on error analysis (MAE, MAPE, RMSE)
Macro definition and multiple parameters
Gnuradio 3.9 using OOT custom module problem record
Gnuradio3.9.4 create OOT module instances
Led serial communication
Kafka connect synchronizes Kafka data to MySQL
Guojingxin center "APEC investment +": some things about the Internet sector today | observation on stabilizing strategic industrial funds
npm 内部拆分模块
FIR filter of IQ signal after AD phase discrimination
STM32GPIO口的工作原理
液压旋转接头的使用事项
Leetcode notes No.7