当前位置:网站首页>C#/VB.NET 给PDF文档添加文本/图像水印
C#/VB.NET 给PDF文档添加文本/图像水印
2022-07-06 10:41:00 【InfoQ】
给PDF文档添加文本水印
- 创建 PdfDocument 对象并用PdfDocument.LoadFromFile()方法加载示例文档。
- 用PdfFontBase.MeasureString()方法设置水印文字和测量文字大小。
- 浏览文档中的所有页面。
- 使用 PdfPageBase.Canvas.TraslateTransform()方法将某个页面的坐标系平移指定坐标,使用 PdfPageBase.Canvas.RotateTransform() 方法将坐标系逆时针旋转 45 度。
- 使用PdfPageBase.Canvas.DrawString()方法在页面上绘制水印文字。
- 用PdfDocument.SaveToFile()方法保存为PDF文件。
using Spire.Pdf;
using Spire.Pdf.Graphics;
using System.Drawing;
namespace AddTextWatermarkToPdf
{
class Program
{
static void Main(string[] args)
{
//创建 PdfDocument 对象
PdfDocument pdf = new PdfDocument();
//加载PDF文档
pdf.LoadFromFile("Sample.pdf");
//创建PdfTrueTypeFont对象
PdfTrueTypeFont font = new PdfTrueTypeFont(new Font("Arial", 50f), true);
//设置水印文本
string text = "CONFIDENTIAL";
//测量文本尺寸
SizeF textSize = font.MeasureString(text);
//计算两个偏移变量的值,用于计算坐标系的平移量
float offset1 = (float)(textSize.Width * System.Math.Sqrt(2) / 4);
float offset2 = (float)(textSize.Height * System.Math.Sqrt(2) / 4);
//浏览文档中的所有页面。
foreach (PdfPageBase page in pdf.Pages)
{
//设置页面透明度
page.Canvas.SetTransparency(0.8f);
//通过指定坐标平移坐标系 page.Canvas.TranslateTransform(page.Canvas.Size.Width / 2 - offset1 - offset2, page.Canvas.Size.Height / 2 + offset1 - offset2);
//将坐标系逆时针旋转 45 度
page.Canvas.RotateTransform(-45);
//在页面上绘制水印文字
page.Canvas.DrawString(text, font, PdfBrushes.DarkGray, 0, 0);
}
//保存修改为新文件
pdf.SaveToFile("TextWatermark.pdf");
}
}
}
Imports Spire.Pdf
Imports Spire.Pdf.Graphics
Imports System.Drawing
Namespace AddTextWatermarkToPdf
Class Program
Private Shared Sub Main(ByVal args() As String)
'创建 PdfDocument 对象
Dim pdf As PdfDocument = New PdfDocument
'加载PDF文档
pdf.LoadFromFile("Sample.pdf")
'创建PdfTrueTypeFont 对象
Dim font As PdfTrueTypeFont = New PdfTrueTypeFont(New Font("Arial", 50!), true)
'设置水印文本
Dim text As String = "CONFIDENTIAL"
'测量文本尺寸
Dim textSize As SizeF = font.MeasureString(text)
'计算两个偏移变量的值,用于计算坐标系的平移量
Dim offset1 As Single = CType((textSize.Width _
* (System.Math.Sqrt(2) / 4)),Single)
Dim offset2 As Single = CType((textSize.Height _
* (System.Math.Sqrt(2) / 4)),Single)
'浏览文档中的所有页面。
For Each page As PdfPageBase In pdf.Pages
'设置页面透明度
page.Canvas.SetTransparency(0.8!)
'通过指定坐标平移坐标系
page.Canvas.TranslateTransform(((page.Canvas.Size.Width / 2) _
- (offset1 - offset2)), ((page.Canvas.Size.Height / 2) _
+ (offset1 - offset2)))
'将坐标系逆时针旋转 45 度
page.Canvas.RotateTransform(-45)
'在页面上绘制水印文字
page.Canvas.DrawString(text, font, PdfBrushes.DarkGray, 0, 0)
Next
'保存修改为新文件
pdf.SaveToFile("TextWatermark.pdf")
End Sub
End Class
End Namespace
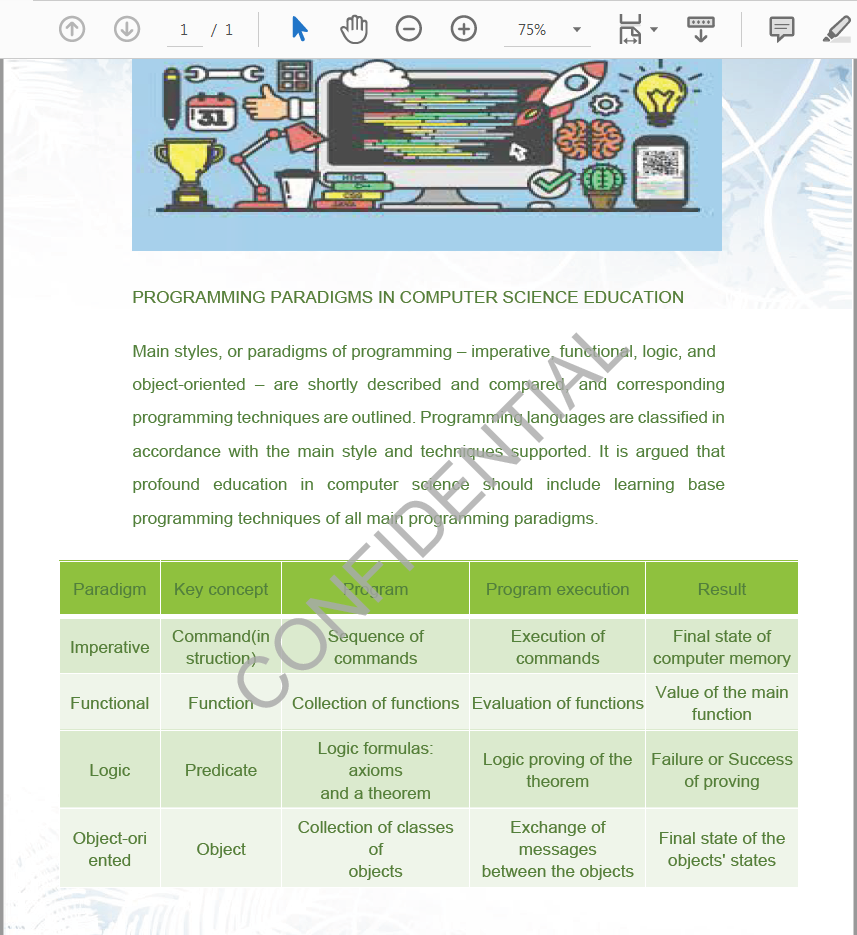
向PDF添加多行文本水印
- 创建PDF文档并用PdfDocument. LoadFromFile()方法加载文件。
- 获取第一页。
- 绘制文字水印。 使用 PdfCanvas.TranslateTransform() 方法设置文本大小。 使用PdfCanvas.RotateTransform()方法设置字体角度。并使用 PdfCanvas.DrawString() 方法绘制您想要的文本内容。
- 使用 PdfDocument.SaveToFile() 方法将文档保存到新的PDF文件。
using System.Drawing;
using Spire.Pdf;
using Spire.Pdf.Graphics;
namespace TextWaterMark
{
class Program
{
static void Main(string[] args)
{
//创建PDF文档并加载文件
PdfDocument doc = new PdfDocument();
doc.LoadFromFile("Sample1.pdf");
//获取第一页
PdfPageBase page = doc.Pages[0];
//绘制文字水印
PdfTilingBrush brush
= new PdfTilingBrush(new SizeF(page.Canvas.ClientSize.Width / 2, page.Canvas.ClientSize.Height / 3));
brush.Graphics.SetTransparency(0.3f);
brush.Graphics.Save();
brush.Graphics.TranslateTransform(brush.Size.Width / 2, brush.Size.Height / 2);
brush.Graphics.RotateTransform(-45);
brush.Graphics.DrawString("internal use",
new PdfFont(PdfFontFamily.Helvetica, 24), PdfBrushes.Violet, 0, 0,
new PdfStringFormat(PdfTextAlignment.Center));
brush.Graphics.Restore();
brush.Graphics.SetTransparency(1);
page.Canvas.DrawRectangle(brush, new RectangleF(new PointF(0, 0), page.Canvas.ClientSize));
//保存为PDF文件
doc.SaveToFile("TextWaterMark1.pdf");
}
}
}
Imports System.Drawing
Imports Spire.Pdf
Imports Spire.Pdf.Graphics
Namespace TextWaterMark
Class Program
Private Shared Sub Main(ByVal args() As String)
'创建PDF文档并加载文件
Dim doc As PdfDocument = New PdfDocument
doc.LoadFromFile("Sample1.pdf")
'获取第一页
Dim page As PdfPageBase = doc.Pages(0)
'绘制文字水印
Dim brush As PdfTilingBrush = New PdfTilingBrush(New SizeF((page.Canvas.ClientSize.Width / 2), (page.Canvas.ClientSize.Height / 3)))
brush.Graphics.SetTransparency(0.3!)
brush.Graphics.Save
brush.Graphics.TranslateTransform((brush.Size.Width / 2), (brush.Size.Height / 2))
brush.Graphics.RotateTransform(-45)
brush.Graphics.DrawString("internal use", New PdfFont(PdfFontFamily.Helvetica, 24), PdfBrushes.Violet, 0, 0, New PdfStringFormat(PdfTextAlignment.Center))
brush.Graphics.Restore
brush.Graphics.SetTransparency(1)
page.Canvas.DrawRectangle(brush, New RectangleF(New PointF(0, 0), page.Canvas.ClientSize))
'保存为PDF文件
doc.SaveToFile("TextWaterMark1.pdf")
End Sub
End Class
End Namespace
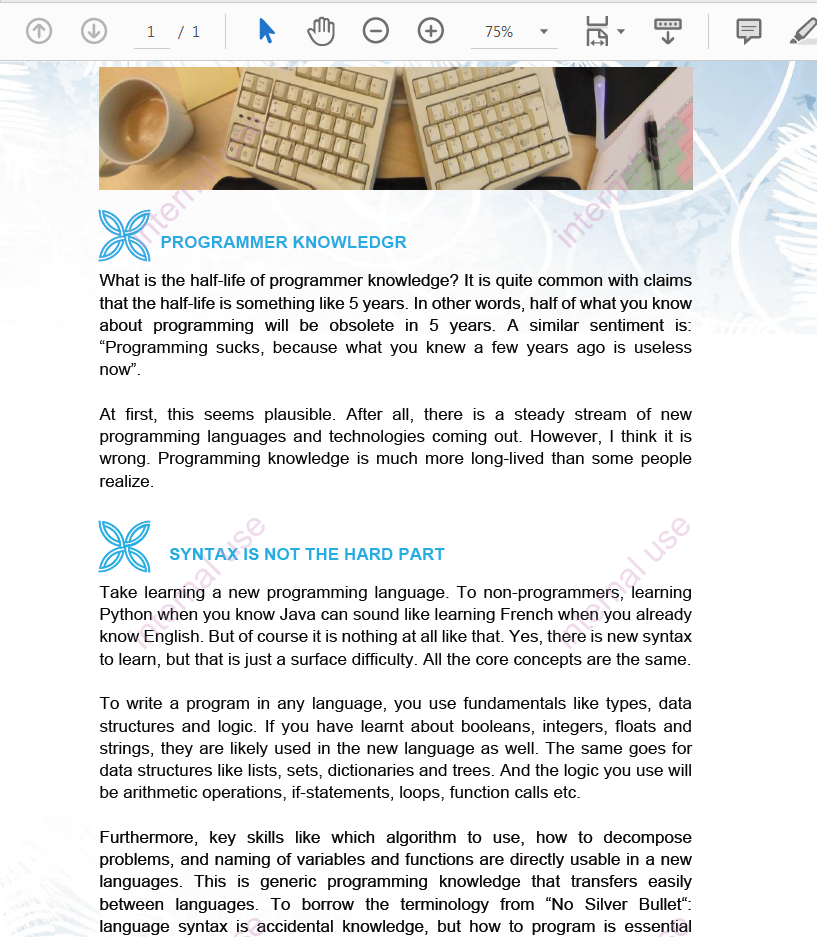
给PDF添加图像水印
- 创建一个PdfDocument 对象并使用PdfDocument.LoadFromFile() 方法加载一个示例PDF 文档。
- 使用 Image.FromFile() 方法加载图像。
- 获取图片尺寸。
- 循环浏览文档中的页面,通过PdfDocument.Pages() 属性获取具体页面。
- 通过PdfPageBase.BackgroundImage 属性设置图片为当前页面的水印图片。通过 PdfPageBase.BackgroundRegion 属性设置图像位置和大小。
- 使用 PdfDocument.SaveToFile() 方法将文档保存到文件中。
using Spire.Pdf;
using System.Drawing;
namespace AddImageWatermark
{
class Program
{
static void Main(string[] args)
{
//创建一个PdfDocument 对象
PdfDocument document = new PdfDocument();
//加载一个示例PDF 文档
document.LoadFromFile("sample.pdf");
//加载图像
Image image = Image.FromFile("logo.png");
//获取图片尺寸
int imgWidth = image.Width;
int imgHeight = image.Height;
//浏览文档中的页面
for (int i = 0; i < document.Pages.Count; i++)
{
//获取页面宽高
float pageWidth = document.Pages[i].ActualSize.Width;
float pageHeight = document.Pages[i].ActualSize.Height;
//设置背景不透明度
document.Pages[i].BackgroudOpacity = 0.3f;
//设置图片为当前页面的水印图片
document.Pages[i].BackgroundImage = image;
//将背景图片放在页面的中心
Rectangle rect = new Rectangle((int)(pageWidth - imgWidth) / 2, (int)(pageHeight - imgHeight) / 2, imgWidth, imgHeight);
document.Pages[i].BackgroundRegion = rect;
}
//将文档保存为PDF文件
document.SaveToFile("AddImageWatermark.pdf");
document.Close();
}
}
}
Imports Spire.Pdf
Imports System.Drawing
Namespace AddImageWatermark
Class Program
Private Shared Sub Main(ByVal args() As String)
'创建一个PdfDocument 对象
Dim document As PdfDocument = New PdfDocument
'加载一个示例PDF 文档
document.LoadFromFile("sample.pdf")
'加载图像
Dim image As Image = Image.FromFile("logo.png")
'获取图片尺寸
Dim imgWidth As Integer = image.Width
Dim imgHeight As Integer = image.Height
'浏览文档中的页面
Dim i As Integer = 0
Do While (i < document.Pages.Count)
'获取页面宽高
Dim pageWidth As Single = document.Pages(i).ActualSize.Width
Dim pageHeight As Single = document.Pages(i).ActualSize.Height
'设置背景不透明度
document.Pages(i).BackgroudOpacity = 0.3!
'设置图片为当前页面的水印图片
document.Pages(i).BackgroundImage = image
'将背景图片放在页面的中心
Dim rect As Rectangle = New Rectangle((CType((pageWidth - imgWidth),Integer) / 2), (CType((pageHeight - imgHeight),Integer) / 2), imgWidth, imgHeight)
document.Pages(i).BackgroundRegion = rect
i = (i + 1)
Loop
'将文档保存为PDF文件
document.SaveToFile("AddImageWatermark.pdf")
document.Close
End Sub
End Class
End Namespace
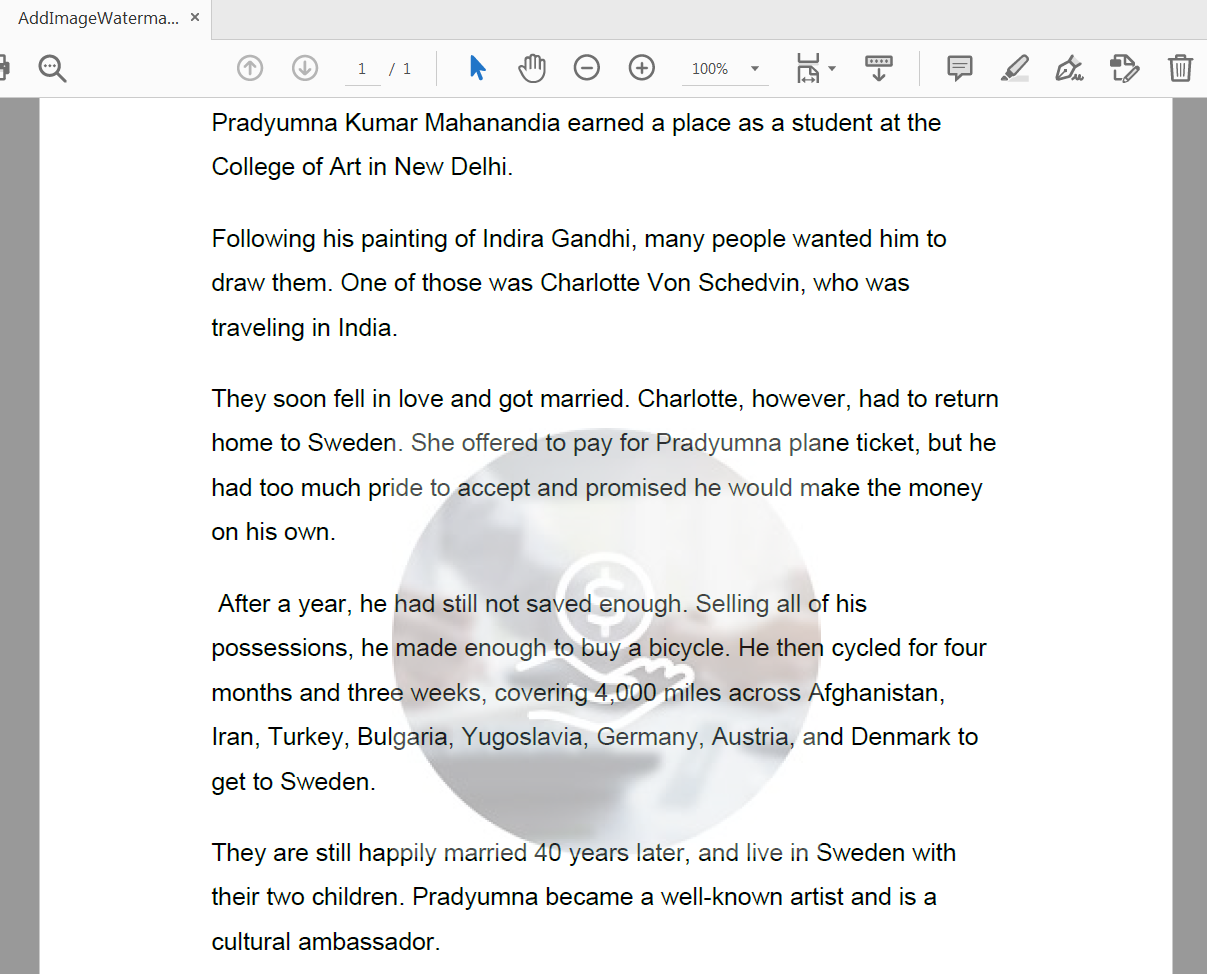
边栏推荐
- 巨杉数据库首批入选金融信创解决方案!
- POJ 2208 已知边四面体六个长度,计算体积
- 【LeetCode第 300 场周赛】
- 虚拟机VirtualBox和Vagrant安装
- [Sun Yat sen University] information sharing of postgraduate entrance examination and re examination
- Alibaba cloud international ECS cannot log in to the pagoda panel console
- celery最佳实践
- Grafana 9.0 is officially released! It's the strongest!
- 287. 寻找重复数
- POJ 2208 six lengths of tetrahedron are known, and the volume is calculated
猜你喜欢
虚拟机VirtualBox和Vagrant安装
std::true_ Type and std:: false_ type
2022-2024年CIFAR Azrieli全球学者名单公布,18位青年学者加入6个研究项目
Recommend easy-to-use backstage management scaffolding, everyone open source
巨杉数据库首批入选金融信创解决方案!
阿里云国际版ECS云服务器无法登录宝塔面板控制台
递归的方式
287. 寻找重复数
[Sun Yat sen University] information sharing of postgraduate entrance examination and re examination
Splay
随机推荐
Excellent open source fonts for programmers
[.Net core] solution to error reporting due to too long request length
Declval of template in generic programming
A method of sequentially loading Unity Resources
Using block to realize the traditional values between two pages
Top command details
Penetration test information collection - CDN bypass
用友OA漏洞学习——NCFindWeb 目录遍历漏洞
287. Find duplicates
Prophet模型的简介以及案例分析
2022/02/12
Declval (example of return value of guidance function)
UDP protocol: simple because of good nature, it is inevitable to encounter "city can play"
Redis的五种数据结构
Comparative examples of C language pointers *p++, * (p++), * ++p, * (++p), (*p) + +, +(*p)
Recursive way
從交互模型中蒸餾知識!中科大&美團提出VIRT,兼具雙塔模型的效率和交互模型的性能,在文本匹配上實現性能和效率的平衡!...
Some understandings of tree LSTM and DGL code implementation
小程序在产业互联网中的作用
2022 Summer Project Training (II)